Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial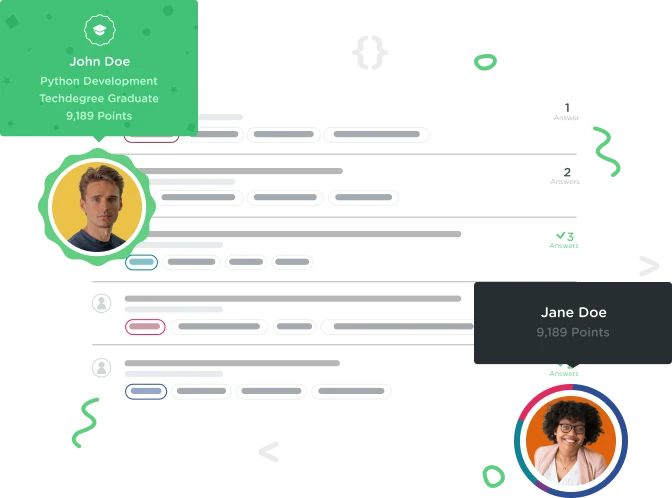

Tarek El jallouly
30 PointsAsp.net Identity to an Existing Database
Hello,
I have one aplication that use custom authentication, yet i need to implement asp.net Identity, i have some problems to do this.
My user table : Utente (IDUtente, Username, .......)
i Deleted Utente table and i add all columns to AspNetUsers, the new table AspNetUsers has columns :
[Id]
,[Email]
,[EmailConfirmed]
,[PasswordHash]
,[SecurityStamp]
,[PhoneNumber]
,[PhoneNumberConfirmed]
,[TwoFactorEnabled]
,[LockoutEndDateUtc]
,[LockoutEnabled]
,[AccessFailedCount]
,[UserName]
,[IDUtente]
,[Password]
,[BackOfficeUserID]
After that i renamed AspNetUsers to Utente, because i want that my application use always 'Utente' name.
After i created all classes :
public class AppDbContext : IdentityDbContext<User> { public AppDbContext() : base("DefaultConnection", throwIfV1Schema: false) { }
protected override void OnModelCreating(DbModelBuilder modelBuilder)
{
Database.SetInitializer<AppDbContext>(null);
base.OnModelCreating(modelBuilder);
modelBuilder.Entity<User>().ToTable("Utente");
}
public static AppDbContext Create()
{
return new AppDbContext();
}
}
public class AppUserManager : UserManager<User> { public AppUserManager(IUserStore<User> store) : base(store) { }
public static AppUserManager Create(IdentityFactoryOptions<AppUserManager> options, IOwinContext context)
{
var manager = new AppUserManager(new UserStore<User>(context.Get<AppDbContext>()));
// Configure validation logic for usernames
manager.UserValidator = new UserValidator<User>(manager)
{
AllowOnlyAlphanumericUserNames = false,
RequireUniqueEmail = true
};
// Configure validation logic for passwords
manager.PasswordValidator = new PasswordValidator
{
RequiredLength = 6,
RequireNonLetterOrDigit = true,
RequireDigit = true,
RequireLowercase = true,
RequireUppercase = true,
};
// Configure ApplicationUser lockout defaults
manager.UserLockoutEnabledByDefault = true;
manager.DefaultAccountLockoutTimeSpan = TimeSpan.FromMinutes(5);
manager.MaxFailedAccessAttemptsBeforeLockout = 5;
// Register two factor authentication providers. This application uses Phone and Emails as a step of receiving a code for verifying the ApplicationUser
// You can write your own provider and plug it in here.
manager.RegisterTwoFactorProvider("Phone Code", new PhoneNumberTokenProvider<User>
{
MessageFormat = "Your security code is {0}"
});
manager.RegisterTwoFactorProvider("Email Code", new EmailTokenProvider<User>
{
Subject = "Security Code",
BodyFormat = "Your security code is {0}"
});
manager.EmailService = new EmailService();
manager.SmsService = new SmsService();
var dataProtectionProvider = options.DataProtectionProvider;
if (dataProtectionProvider != null)
{
manager.UserTokenProvider =
new DataProtectorTokenProvider<User>(dataProtectionProvider.Create("ASP.NET Identity"));
}
return manager;
}
}
public class AppSignInManager : SignInManager<User, string> { public AppSignInManager(AppUserManager userManager, IAuthenticationManager authenticationManager) : base(userManager, authenticationManager) { }
public override Task<ClaimsIdentity> CreateUserIdentityAsync(User user)
{
return user.GenerateUserIdentityAsync((AppUserManager)UserManager);
}
public static AppSignInManager Create(IdentityFactoryOptions<AppSignInManager> options, IOwinContext context)
{
return new AppSignInManager(context.GetUserManager<AppUserManager>(), context.Authentication);
}
}
in the App_Start, is created Startup class :
Public Sub ConfigureAuth(app As IAppBuilder)
app.CreatePerOwinContext(AddressOf AppDbContext.Create)
app.CreatePerOwinContext(Of AppUserManager)(AddressOf AppUserManager.Create)
app.CreatePerOwinContext(Of AppSignInManager)(AddressOf AppSignInManager.Create)
app.UseCookieAuthentication(New CookieAuthenticationOptions With {
.AuthenticationType = DefaultAuthenticationTypes.ApplicationCookie,
.Provider = New CookieAuthenticationProvider With {
.OnValidateIdentity = SecurityStampValidator.OnValidateIdentity(Of AppUserManager, Sportrick.Model.User)(
validateInterval:=TimeSpan.FromMinutes(30),
regenerateIdentity:=Function(manager, user) user.GenerateUserIdentityAsync(manager))
}
})
app.UseExternalSignInCookie(DefaultAuthenticationTypes.ExternalCookie)
app.UseTwoFactorSignInCookie(DefaultAuthenticationTypes.TwoFactorCookie, TimeSpan.FromMinutes(5))
app.UseTwoFactorRememberBrowserCookie(DefaultAuthenticationTypes.TwoFactorRememberBrowserCookie)
End Sub
In the End, in accountController :
<HttpPost> <AllowAnonymous> Public Async Function LogOn(ByVal model As LogOnViewModel, ByVal returnUrl As String) As Task(Of ActionResult) If Not ModelState.IsValid Then Return View(model) End If
Dim result = Await SignInManager.PasswordSignInAsync(model.Username, model.Password, model.RememberMe, shouldLockout:=False)
Select Case result
Case SignInStatus.Success
Return RedirectToLocal(returnUrl)
Case SignInStatus.LockedOut
Return View("Lockout")
Case SignInStatus.RequiresVerification
Return RedirectToAction("SendCode", New With {Key .ReturnUrl = returnUrl, Key .RememberMe = model.RememberMe})
Case Else
ModelState.AddModelError("", "Invalid login attempt.")
Return View(model)
End Select
End Function
Error Login :
The foreign key component 'UserId' is not a declared property on type 'Login'. Verify that it has not been explicitly excluded from the model and that it is a valid primitive property.
UserId is the Key of User entity (i have mapping in EDMX model tra Entity User <-> Table Utente)