Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial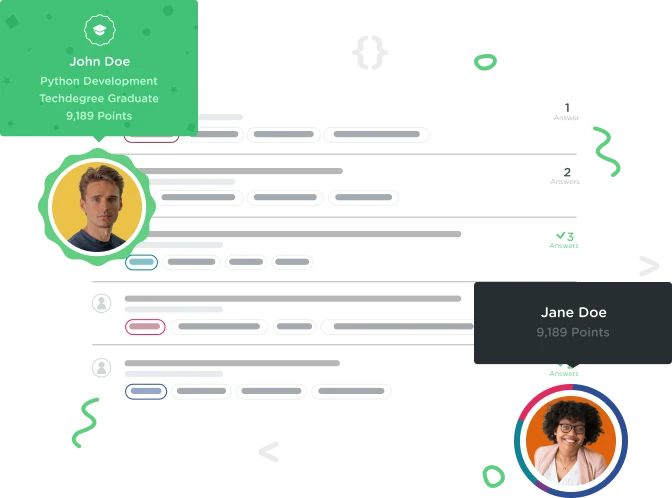

Larry Singleton
18,946 PointsASP.NET MVC FORMS
I can't figure out what I'm doing wrong.
Link: https://teamtreehouse.com/library/aspnet-mvc-forms/creating-a-basic-form/adding-a-post-action-method
Question:
Challenge Task 1 of 1
In IssuesController.cs add another action method named Report to handle HTTP posts from the form in the Report.cshtml view.
Decorate the action method with an attribute so that MVC will only route POST requests to it.
Add a total of six parameters that match the form field names in the Report.cshtml view file in order to capture posted values from the form. Remember to change the first letter of each form field to lowercase (i.e. "Name" to "name").
Use the string data type for each parameter.
For now, in the action method, just return a call to the View method.
IssuesController.cs file
using System.Web.Mvc;
namespace IssueReporter.Controllers
{
public class IssuesController : Controller
{
public ActionResult Report()
{
return View();
}
}
}
Report.cshtml file:
@{
ViewBag.Title = "Report an Issue";
}
<h2>@ViewBag.Title</h2>
<form method="post">
<div>
<label for="Name">Name</label>
<input type="text" id="Name" name="Name" />
</div>
<div>
<label for="Email">Email</label>
<input type="text" id="Email" name="Email" />
</div>
<div>
<label for="DepartmentId">Department</label>
<input type="text" id="DepartmentId" name="DepartmentId" />
</div>
<div>
<label for="Severity">Severity</label>
<input type="text" id="Severity" name="Severity" />
</div>
<div>
<label for="Reproducible">Reproducible</label>
<input type="text" id="Reproducible" name="Reproducible" />
</div>
<div>
<label for="DescriptionOfProblem">Description of Problem</label>
<textarea id="DescriptionOfProblem" name="DescriptionOfProblem"></textarea>
</div>
<button type="submit">Save</button>
</form>
One of my answers:
IssuesController.cs:
using System.Web.Mvc;
namespace IssueReporter.Controllers
{
public class IssuesController : Controller
{
public ActionResult Report()
{
return View();
}
[HttpPost]
public ActionResult Add(string name, string email,
string departmentId, string severity, string reproducible,
string descriptionofproblem)
{
viewBag.Name = name;
viewBag.Email = email;
viewBag.DepartmentId = departmentId;
viewBag.Severity = severity;
viewBag.Reproducible = reproducible;
return View();
}
}
}
Report.cshtml
@{ ViewBag.Title = "Report an Issue"; }
<h2>@ViewBag.Title</h2>
<form method="post">
<div>
<label for="Name">Name</label>
<input type="text" id="Name" name="Name" value="@viewBag.Name" />
</div>
<div>
<label for="Email">Email</label>
<input type="text" id="Email" name="Email" value="@viewBag.Email" />
</div>
<div>
<label for="departmentId">Department</label>
<input type="text" id="DepartmentId" name="DepartmentId" value="@viewBag.DepartmentId" />
</div>
<div>
<label for="severity">Severity</label>
<input type="text" id="Severity" name="Severity" value="@viewBag.Severity" />
</div>
<div>
<label for="reproducible">Reproducible</label>
<input type="text" id="Reproducible" name="Reproducible" value="@viewBag.Reproducible" />
</div>
<div>
<label for="descriptionOfProblem">Description of Problem</label>
<textarea id="DescriptionOfProblem" name="DescriptionOfProblem"> </textarea>
</div>
<button type="submit">Save</button>
</form>
I've followed the video 4-5 times now, it's not working for me.
```IssuesController.cs
using System.Web.Mvc;
namespace IssueReporter.Controllers
{
public class IssuesController : Controller
{
public ActionResult Report()
{
return View();
}
[HttpPost]
public ActionResult Add(string name, string email,
string departmentId, string severity, string reproducible,
string descriptionofproblem)
{
return View();
}
}
}
@{
ViewBag.Title = "Report an Issue";
}
<h2>@ViewBag.Title</h2>
<form method="post">
<div>
<label for="Name">Name</label>
<input type="text" id="Name" name="Name" />
</div>
<div>
<label for="Email">Email</label>
<input type="text" id="Email" name="Email" />
</div>
<div>
<label for="DepartmentId">Department</label>
<input type="text" id="DepartmentId" name="DepartmentId" />
</div>
<div>
<label for="Severity">Severity</label>
<input type="text" id="Severity" name="Severity" />
</div>
<div>
<label for="Reproducible">Reproducible</label>
<input type="text" id="Reproducible" name="Reproducible" />
</div>
<div>
<label for="DescriptionOfProblem">Description of Problem</label>
<textarea id="DescriptionOfProblem" name="DescriptionOfProblem"></textarea>
</div>
<button type="submit">Save</button>
</form>
4 Answers

James Churchill
Treehouse TeacherLarry,
Are you getting an error message? Also, remembering that C# is a case sensitive language, double check the casing of your action method parameter names.
Thanks ~James

Larry Singleton
18,946 PointsHere is the error, guess I should have posted this as well. And, thanks for responding.
IssuesController.cs(42,9): error CS0103: The name `viewBag' does not exist in the current context
IssuesController.cs(43,9): error CS0103: The name `viewBag' does not exist in the current context
IssuesController.cs(44,9): error CS0103: The name `viewBag' does not exist in the current context
IssuesController.cs(45,9): error CS0103: The name `viewBag' does not exist in the current context
IssuesController.cs(46,9): error CS0103: The name `viewBag' does not exist in the current context
Compilation failed: 5 error(s), 0 warnings

James Churchill
Treehouse TeacherLarry,
Ah... that helps. The property name is actually ViewBag
not viewBag
.
https://msdn.microsoft.com/en-us/library/system.web.mvc.controllerbase.viewbag(v=vs.118).aspx
Try making that change and see what error happens next :)
Thanks ~James

Larry Singleton
18,946 PointsBreaking it down to one step at a time, I have this now in the IssuesController.cs file
using System.Web.Mvc;
namespace IssueReporter.Controllers
{
public class IssuesController : Controller
{
public ActionResult Report()
{
return View();
}
[HttpPost]
public ActionResult Add(string name, string email,
string departmentId, string severity, string reproducible,
string descriptionofproblem)
{
ViewBag.Date = name;
ViewBag.Email = email;
ViewBag.DepartmentId = departmentId;
ViewBag.Severity = severity;
ViewBag.Reproducible = reproducible;
return View();
}
}
}
It's giving me this error:
Bummer! Did you declare a method named Report in IssuesController?
I'm not seeing the problem. I've left the report.cshtml file to its unaltered original state and will work on it when I get the IssuesController.cs file fixed. I stepped away from this to hopefully give me enough time to undo any brain farts that may be going on. Tonight I will watch the videos again and put more effort into it.
Again, thanks for responding, Larry

Larry Singleton
18,946 PointsI finally figured it out, thanks for the guidance.