Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial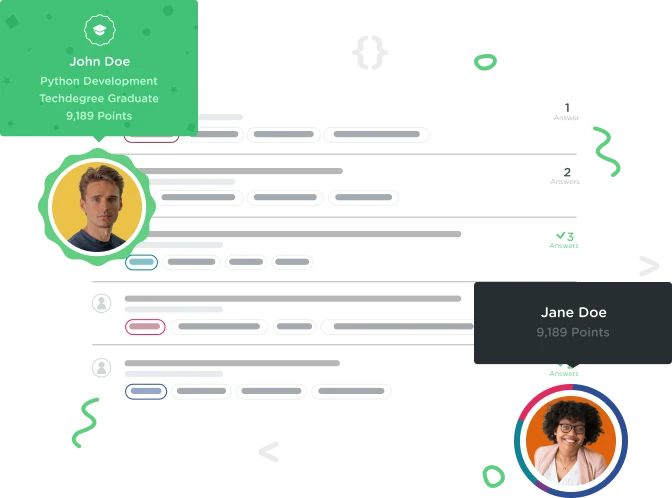

Kris Larsen
8,015 PointsAssign key to constant named applePie
Let's pretend a customer has ordered an ice cream. Retrieve the value for apple pie using the correct key and assign it to a constant named applePie.
// Enter your code below
var iceCream = [
"CC": "Chocolate Chip",
"AP": "Apple Pie",
"PB": "Peanut Butter"
]
iceCream.updateValue("Rocky Road", forKey: "RR")
iceCream.removeValueForKey("AP")
let applePie = "AP"
4 Answers
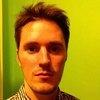
Brendan Whiting
Front End Web Development Techdegree Graduate 84,735 PointsThey don't want you to remove Apple pie from the dictionary permanently, that's what the removeValueForKey function does. They just want you to look up the value for the key, leaving it intact, which uses this syntax: iceCream["AP"]
Also they want you to put that value into the applePie
constant programmatically. And what should ultimately end up inside applePie
should be the string "Apple Pie" not "AP". The code should be this:
let applePie = iceCream["AP"]
This looks into the iceCream dictionary to see the value for the key "AP", and then it takes that value and puts it inside of the constant applePie. (And remember to get rid of the line above with removeValueForKey otherwise there won't be an apple pie in there to look for).

ALAN SALDANA
357 Pointsthis is not working , anyway u try. using what teacher tells u on practice, and there is no help . only bugging EMAILS, TELLING ME HOW GREAT IM DOPING BUT THERE IS NOT SUPPORT.
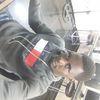
Kellington Chidza
866 Pointslet applePie = iceCream["AP"]

Rich Braymiller
7,119 Pointssee now this doesn't make sense at all...in the Example during the video was //read from dictionary
airportCodes["LGA"]
the var airportCodes was declared earlier
So why doesn't this code work for the Code Challenge????????
var iceCream: = [ "CC": "Chocolate Chip", "AP": "Apple Pie", "PB": "Peanut Butter" ]
iceCream.updateValue("Rocky Road", forKey: "RR")
iceCream ["AP"]

renato pozagn
475 Pointsit does work but the compiler doesnt recognise it ,, why i ahwe no idea
Emmanuel Darmon
6,115 PointsEmmanuel Darmon
6,115 PointsThanks for your help Brendan. But I do not understand why it doesn't work this way:
I assigned to the constant applePie the value I'm deleting from the dictionary. Isn't it?
Brendan Whiting
Front End Web Development Techdegree Graduate 84,735 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,735 PointsThink of your iceCream dictionary as being the menu. You want to look up the right item on the menu using its key. But you don't want to permanently delete the item from the menu after someone has ordered it once.
The removeValueForKey() function deletes the item at that key and also returns it. The code works, it's just not good business, and the challenge won't allow you to do that. It wants you to use a method that doesn't delete it like the one I mentioned.
Try putting your code into a Swift Playground in Xcode, and add a line to check for the contents of the iceCream dictionary at the end, and you'll see that it's missing Apple Pie.