Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial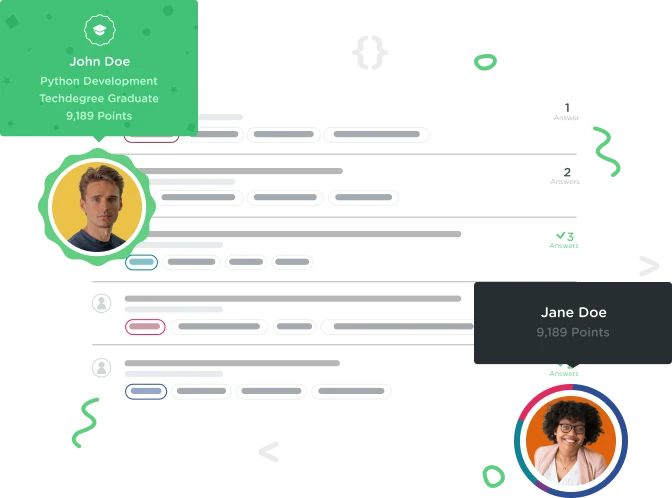
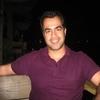
Renan A
4,425 PointsAssigning an object from a shoppingList NSArray as a shoppingCart NSString value
Can't figure out what's wrong
#import "UIViewController.h"
@interface ViewController : UIViewController
@property (strong, nonatomic) NSString *shoppingCart;
@property (strong, nonatomic) NSArray *shoppingList;
@end
#import "ViewController.h"
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Add your code below!
self.shoppingList = [[NSArray alloc]initWithObjects: @"toothpaste",@"bread",@"eggs",nil];
shoppingCart = [shoppingList objectAtIndex:2];
}
@end
3 Answers
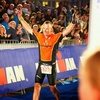
Steve Hunter
57,712 PointsHi Renan,
You're very close.
You need to use self
on the array variable name and the NSString too:
self.shoppingList = [[NSArray alloc]initWithObjects: @"toothpaste",@"bread",@"eggs",nil];
self.shoppingCart = [self.shoppingList objectAtIndex: 2];
I hope that helps,
Steve.

Anjali Pasupathy
28,883 PointsYou're nearly there! You just need to call self.shoppingCart and self.shoppingList in the last line of viewDidLoad:
self.shoppingCart = [self.shoppingList objectAtIndex:2];
I hope this helps!
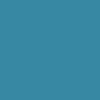
Sai Kiran Dasika
Courses Plus Student 7,278 PointsWhat you have done is correct except you made a silly mistake by not adding the self keyword before the shopping cart as it's a property of the ViewController class. The following code should work properly
self.shoppingCart = [self.shoppingList objectAtIndex:2];