Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial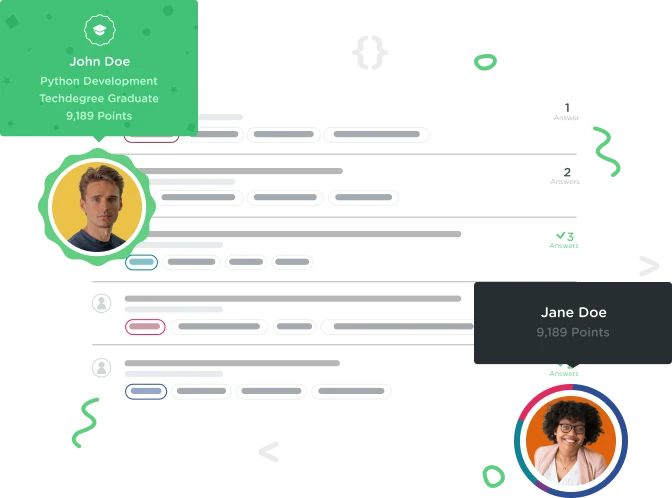
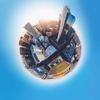
Joel Crouse
4,727 PointsAssigning array of objects to an object property
Basically after reviewing the code below you will see that I have two structs one for Quotes and the other for People who said them. The Person struct has a property assigning that person all his/her array of quotes. I'm not sure how to do this and its kind of hard to explain but I'm sure you'll get what I'm trying to accomplish. PS: Read the note in the last function.
struct Quote {
let quote: String
let attribution: String
let image: UIImage
}
struct Person {
let name: String
let quotes: [Quote]
}
func createQuotes() -> [Quote] {
var quotes = [Quote]()
let q0 = Quote(quote: "All that glitters is not gold", attribution: "William Shakespeare", image: #imageLiteral(resourceName: "william_shakespeare"))
let q1 = Quote(quote: "Hell is empty and all the devils are here.", attribution: "William Shakespeare", image: #imageLiteral(resourceName: "william_shakespeare"))
let q2 = Quote(quote: "To thine own self be true, and it must follow, as the night the day, thou canst not then be false to any man.", attribution: "William Shakespeare", image: #imageLiteral(resourceName: "william_shakespeare"))
let q3 = Quote(quote: "Love all, trust a few, do wrong to none.", attribution: "William Shakespeare", image: #imageLiteral(resourceName: "william_shakespeare"))
let q4 = Quote(quote: "What's in a name? That which we call a rose by any other name would smell as sweet.", attribution: "William Shakespeare", image: #imageLiteral(resourceName: "william_shakespeare"))
quotes.append(q0)
quotes.append(q1)
quotes.append(q2)
quotes.append(q3)
quotes.append(q4)
return quotes
}
func createPeople() -> [Person] {
var people = [Person]()
let quotes = createQuotes()
let williamShakespeare = Person(name: "William Shakespeare", quotes: // how to give Shakespeare his quotes here???)
return people
}
1 Answer

Magnus Hållberg
17,232 PointsI must say that I don't really understand the structure of the code. You have two structs, Person and Quote. Both have a property for a name, Attribution in Quote and name in Person. It seems repetitive. Even so... it looks like you could use the quotes constant you already have since the struct "Person" have the property quotes that takes an array of Quote and that's just what an instance of the createQuotes function is. In the createPeople function I'm guessing you are going to append more people saying brilliant things, much like you do in the createQuotes function. Inside the body of a function you don't have to create an instance to append, you could just do it like this: quotes.append(Quote(quote: "All that glitters is not gold", attribution: "William Shakespeare", image: #imageLiteral(resourceName: "william_shakespeare"))). So that's 5 lines of code you don't have to write.