Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial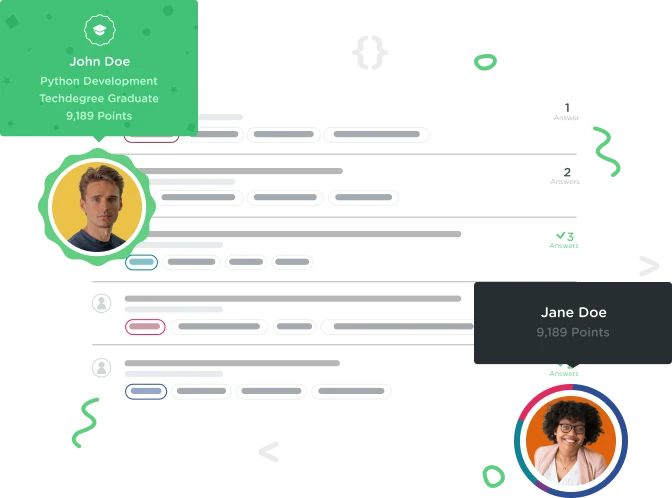

Seth Miller
5,851 PointsAssigning the filter callback to a variable
When you assign the callback to a variable, then pass the variable through the filter function, how does the program know that the element refers to the items in the array? I thought the element would trigger a reference error.
2 Answers

Eduardo Valencia
12,444 PointsYou aren't really passing the parameters; you are passing the function. You can do this because functions are first-class citizens. This means that they can be passed around and assigned to variables, like any other data type. For example:
function toSecondPower(number) {
return number * number;
}
// Assigning to variable without parentheses.
const newVariable = toSecondPower;
console.log(newVariable(5));
// The result is 25, which is just as if you had called the function "toSecondPower" and passed it 5.
Because functions are first class citizens, you can assign them to variables. You can then treat those variables as if they were the function name, and pass them parameters like I did in the line console.log(newVariable(5))
.
That is what happened in the video example you saw. The function was assigned to a variable, and then passed to the filter method. The filter method then passed in each array element to the function, and checked if it returned true. If it did, then it included it in the new array.

Eduardo Valencia
12,444 Pointsnumbers = [1, 2, 3, 4, 5];
numbers.filter(number => number > 2);
Do you mean something like number
in the example above? If so, then it is for the same reason that the example below does not trigger a reference error:
function addFive(number) {
return number + 5;
}
It is because like in this example, number
is a parameter. The programs knows that it refers to the elements in the array because the filter method was built that way. It was built to pass in an array element as an argument to the function you provided it. Because it is a parameter of a function, it does not trigger a reference error.

Seth Miller
5,851 PointsThat makes sense. What I find confusing is that you can take the parameters and assign them to another variable than can be passed to the filter function without calling a reference error.
The part of the video I'm referencing is this (4:14):
const names =[<array of strings>]; const startsWithS = name => name.charAt(0)==='S'; const sNames = names.filter(startsWithS);
Seth Miller
5,851 PointsSeth Miller
5,851 PointsDidn't think about it that way. Thank you for your explanation!