Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial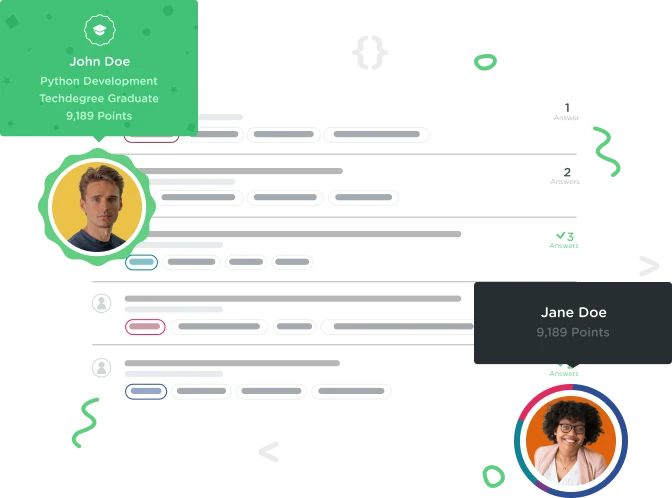

Dolly Shin
9,627 PointsAssist method is correctly called but the "Challenge Task" keeps throwing error
The course "Challenge Task" is throwing an error saying "Did you remember to call the assist method on the CustomerServiceRep. I have correctly used the assist method on the CustomerServiceRep object "csr." There is no compiling error and I even get the right output. I am not sure why it thinks that I am not using the assist method. Please help me understand what is the issue with my code.
public void acceptCustomer(Customer customer) { do { playHoldMusic(); } while(mSupportReps.poll() == null);
CustomerSupportRep csr = mSupportReps.poll();
csr.assist(customer);
mSupportReps.add(csr);
}
import java.util.ArrayDeque;
import java.util.Queue;
public class CallCenter {
Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) {
mSupportReps = queue;
}
public void acceptCustomer(Customer customer) {
do {
playHoldMusic();
} while(mSupportReps.poll() == null);
CustomerSupportRep csr = mSupportReps.poll();
csr.assist(customer);
mSupportReps.add(csr);
System.out.println(csr.getAssistedCustomers());
}
public void playHoldMusic() {
System.out.println("Smooooooth Operator.....");
}
}
import java.util.List;
import java.util.ArrayList;
public class CustomerSupportRep {
private String mName;
private List<Customer> mAssistedCustomers;
public CustomerSupportRep(String name) {
mName = name;
mAssistedCustomers = new ArrayList<Customer>();
}
public void assist(Customer customer) {
System.out.printf("Hello %s, my name is %s, how can I assist you.%n",
customer.getName(),
mName);
System.out.println("...");
System.out.println("Is there anything else I can help you with?");
mAssistedCustomers.add(customer);
}
public List<Customer> getAssistedCustomers() {
return mAssistedCustomers;
}
}
public class Customer {
private String mName;
public Customer(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
3 Answers

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsDolly Shin Steven Parker noob developer
I think I figured it out, see the below code and comments.
public void acceptCustomer(Customer customer) {
/********************************************
* TODO (1)
* Wait until there is an available rep in the queue.
* While there is not one available, playHoldMusic
* HINT: That while assignmentcheck loop syntax we used to
* read files seems pretty similar
********************************************
*/
// you need to work with queue.size() which returns number of Reps in the queue
while (mSupportReps.size() == 0) {
playHoldMusic();
}
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
//to avoid a null pointer exception intialize the Rep here.
CustomerSupportRep csr = mSupportReps.poll();
csr.assist(customer);
//takes off the Rep from queue of available Reps
mSupportReps.poll();
/********************************************
* TODO (3)
* Since the customer support rep is done with
* assisting, put them back into the queue.
********************************************
*/
//adds the Rep back to the queue
mSupportReps.add(csr);
}
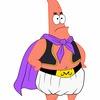
<noob />
17,062 PointsTonnie Fanadez i am a video from this challnage ( i’m at serilization) i will take a look at it when i get home

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 Pointsnoob developer no problem I think I at last go it right :) see my code solution above

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsI also had trouble with this method, I think Queue Interface was the most challenging in this course.
The poll() method just initializes the next available CustomerRep. The method gets the value of the first Rep of available on the Reps queue. The Rep is then pushed out of the Reps Queue and and therefore cannot assist another customer until she is free when pushed back to the Queue by mSupportReps.add(csr) .
I found it as the best way to initialize the Rep and taking her out of the Queue at the same time. When I used .peek() I got an error because peek identifies the Rep to assist but doesn't take her out of the Queue.
I also tried doing CustomerSupportRep csr = new CustomerSupportRep ("rep"); but I kept getting a "BUMMER: did you remember to call the assist method on CustomerSupportRep?"
Cheers.
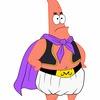
<noob />
17,062 Pointsimport java.util.ArrayDeque;
import java.util.Queue;
///this was my take
public class CallCenter {
Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) {
mSupportReps = queue;
}
//task 1 = First i did a while loop and checked if there there is any "representative" in the queue by checking the queue size, if its 0 there
//is none and i call the playHoldMusic.
public void acceptCustomer(Customer customer) {
CustomerSupportRep csr;
while(mSupportReps.size() == 0)
{
playHoldMusic();
}
//task 2 =task 2 for my opnion was the hardest and i still have question on it but somehow i manage to pass it , i assign csr to result
//of the poll() method which outputs the "representative"?
csr = mSupportReps.poll();
csr.assist(customer);
//task 3 = i put the csr which is a "representative" back to the queue
mSupportReps.add(csr);
}
public void playHoldMusic() {
System.out.println("Smooooooth Operator.....");
}
}
i just dont fully understand this line :
csr = mSupportReps.poll();
what excatly csr contains when i do this?, as far as i know it will retrievses the Rep isnt it?
Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsTonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsI am also getting the same error every time as Dolly Shin . Kindly Steven Parker and noob developer have a look and let us know.