Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial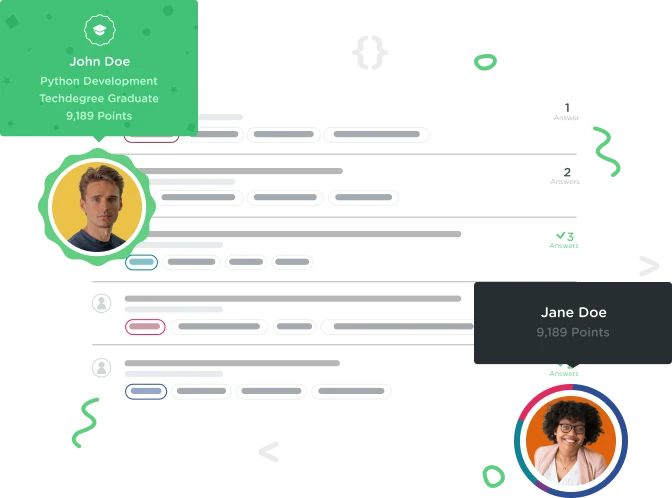

Sridhar Kasukhela
449 PointsAsynchronous execution of callback?
I wrote a simple script (alarm.js) which is supposed to trigger the "alarm" after snoozing for input time.
'use strict';
// set off the alarm
function fn_setOffAlarm() {
console.log('fn_setOffAlarm:'+Date.now()+':Time to wake up!');
}
// get current time continuously in a loop till the current time is equal to sleep time
function fn_snooze(timeInSecs, fn_setOffAlarm) {
var startTime = Date.now();
console.log('fn_snooze:'+startTime+':Start sleeping');
while (startTime+(timeInSecs*1000) >= Date.now()) {
}
// set off the alarm after snoozing long enough
fn_setOffAlarm();
}
console.log('alarm.js:'+Date.now()+':I am going to sleep');
fn_snooze(2,fn_setOffAlarm);
console.log('alarm.js:'+Date.now()+':Enough... now wake up!');
// end of script
The script outputs the following messages:
alarm.js:1500706642192:I am going to sleep
fn_snooze:1500706642195:Start sleeping
fn_setOffAlarm:1500706644196:Time to wake up!
alarm.js:1500706644196:Enough... now wake up!
Since the callbacks are supposed to be executed asynchronously, I was expecting to see the last message before setting off the alarm and then finally the message starting with "fn_setOffAlarm".
Can someone explain why is not so? I executed this script using node $ node alarm.js
Thanks in advance. Sridhar
3 Answers
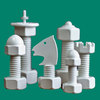
Steven Parker
231,275 PointsThere aren't any asynchronous callbacks in this program.
There's only a function with a synchronous loop that then calls another function.
But you could give it a timer with an asynchronous callback with this modification:
// set a timer to perform a callback when the time elapses
function fn_snooze(timeInSecs, fn_setOffAlarm) {
console.log("fn_snooze:" + Date.now() + ":Start sleeping");
// set off the alarm after snoozing long enough
setTimeout(fn_setOffAlarm, timeInSecs * 1000);
}

Sridhar Kasukhela
449 PointsThanks for the response, Steven.
What you suggested works as you can see below.
alarm.js:1500733048939:I am going to sleep
fn_snooze:1500733048941:Start sleeping
alarm.js:1500733048943:Enough... now wake up!
fn_setOffAlarm:1500733053945:Time to wake up!
How can I make fn_snooze behave like setTimeout so that it executes asynchronously? The idea is to trigger fn_setOffAlarm after so many seconds have elapsed and do that without using setTimeout. I am trying to understand how the callback functions work and since I still trying to get my head around this concept... my question also might not be very clear.
Regards Sridhar
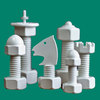
Steven Parker
231,275 PointsI think what you're asking about could be done using aync functions and promises. But this would not be a good application since the original function is so CPU-intensive that anything else might not get run time until it completes.
For this situation setTimeout is the perfect choice. I can't imagine why you want to do this "without using setTimeout".

Sridhar Kasukhela
449 PointsYou are right. This would not be a good application and setTimeout is the perfect choice. Completely agree with you. My idea is to understand how tasks are executed asynchronously in node.js and not to build an application that replicates the functionality of already available functions.
Regards Sridhar
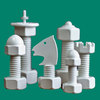
Steven Parker
231,275 PointsMany times you'll find an existing function that uses a callback that will be suited to your purpose.
Also check out this Async function library.