Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial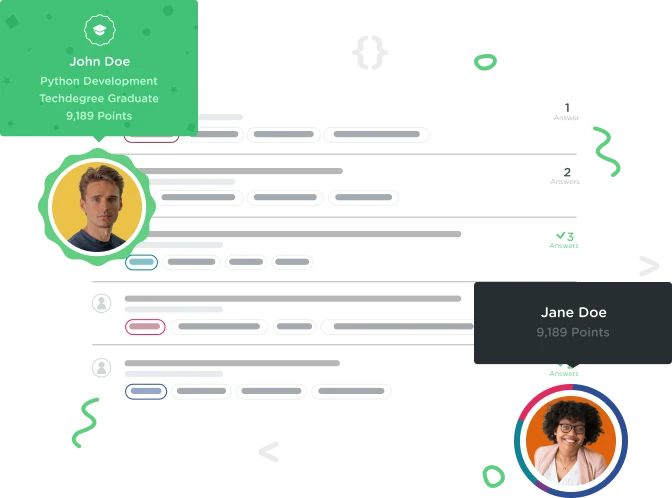
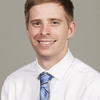
Sean King
5,144 PointsAsynchronous issues with this tutorial
From what I can tell of the results from my console:
<div id="employeeList">
<ul class="bulleted"></ul>
<li class="out"></li>
Aimee
<li class="out"></li>
Amit
<li class="in"></li>
Andrew
...
the file seems to be doing everything out of order. The I assume this is mainly because the loop is completing before the data can be obtained from the JSON file. I'm not really sure. Here's the code:
const xhr = new XMLHttpRequest();
xhr.onreadystatechange = () => {
if(xhr.readyState === 4) {
let employees = JSON.parse(xhr.responseText);
const list = document.querySelector('#employeeList');
list.innerHTML = '<ul class="bulleted">'
for (let i = 0; i < employees.length; i++) {
if (employees[i].inoffice) {
list.innerHTML += '<li class="in">';
} else {
list.innerHTML += '<li class="out">';
}
list.innerHTML += employees[i].name + '</li>'
}
list.innerHTML += '</ul>'
}
};
xhr.open('GET', 'data/employees.json');
xhr.send();
2 Answers

Unsubscribed User
11,402 PointsHi Sean I've refactored your code below. You can think of this exercise as building a string using the data from the object which was made using the data received from the AJAX Request and then finally using the string which you built to change the innerHTML of the list element in the html
const xhr = new XMLHttpRequest();
xhr.onreadystatechange = () => {
if(xhr.readyState === 4) {
let employees = JSON.parse(xhr.responseText);
var listHTML = '<ul class="bulleted">' // create a string
for (let i = 0; i < employees.length; i++) {
if (employees[i].inoffice === true) {
listHTML += '<li class="in">';
} else {
listHTML += '<li class="out">';
}
listHTML += employees[i].name
listHTML += '</li>';
}
listHTML += '</ul>'
document.getElementById('employeeList').innerHTML = listHTML;
console.log(listHTML)
}
};
xhr.open('GET', 'data/employees.json');
xhr.send();
All I've done is remove your list.innerHTML properties as they were unnecessary in building the string we need. I also moved your query selector to the bottom of the function and changed it to a getElementById method and used the innerHTML property to add our string which i renamed listHTML
Hope this helps your understanding! :)

Mohamed El-Damarawy
11,294 PointsWell you're instructing JS to create a <ul> tag inside the div, so by default the tag is closed, instead you can create that as a var and append the <ul> with the <li> tags when you finish looping over all the objects in the arraylist