Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial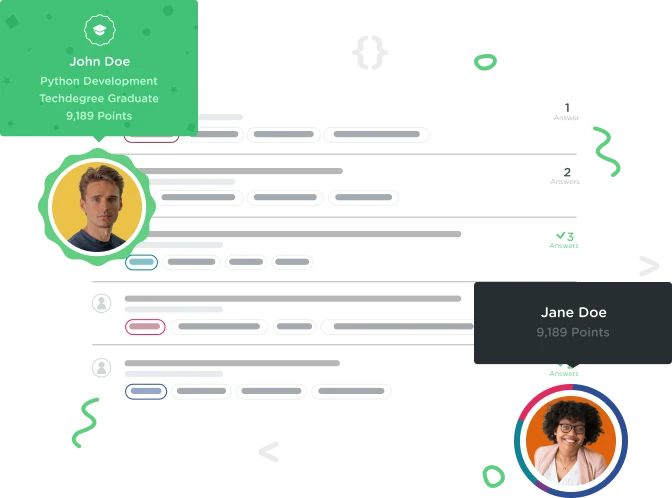

Ryan Carlos
422 PointsAt 01:53... I try to compile and run and get this "cannot find symbol" error.
import java.io.Console;
public class TreeStory {
public static void main(String[] args) {
Console console = System.console();
/* Some terms:
noun - Person, place or thing
verb - An action
adjective - A description used to modify or describe a noun
Enter your amazing code here!
*/
String ageAsString = console.readLine("How old are you? ");
int age = Integer.parseInt(ageAsString);
if (age < 13) {
//insert exit code
console.printf("Sorry, you must be at least 13 to use this program.\n");
System.exit(0);
}
String name = console.readLine("Enter a name: ");
String adjective = console.readLine("Enter an adjective: ");
String noun = console.readLine("Enter a noun: ");
if (noun.equalsIgnoreCase ("dork") || noun.equalsIngoreCase ("jerk")) {
console.printf("That language is not allowed, Exiting. \n\n");
System.exit(0);
}
String adverb = console.readLine("Enter an adverb: ");
String verb = console.readLine("Enter a verb ending with -ing: ");
console.printf("\nYour TreeStory: \n---------------\n");
console.printf("%s is a %s %s. ", name, adjective, noun);
console.printf("They are always %s %s.\n", adverb, verb);
}
}
That's my code but the console error says... on the compile...
javac TreeStory.java
TreeStory.java:23: error: cannot find symbol
if (noun.equalsIgnoreCase("dork") || noun.equalsIngoreCase("jerk")) {
^
symbol: method equalsIngoreCase(String)
location: variable noun of type String
1 error
Need help. Thanks.
4 Answers

Andrew Winkler
37,739 PointsTo be articulate, you made a typo concerning the 'g' and the 'n' in the 2nd equalsIgnoreCase() on line 23. look at the error code closely:
javac TreeStory.java
TreeStory.java:23: error: cannot find symbol
if (noun.equalsIgnoreCase("dork") || noun.equals*Ingore*Case("jerk")) { ^
symbol: method equals*Ingore*Case(String)
location: variable noun of type String
1 error

Andrew Winkler
37,739 PointsTry this instead:
import java.io.Console;
pulblic class TreeStory{
public static void main(String[] args) {
Console console = System.console();
/* Some terms:
noun - Person, place or thing
verb - An action
adjective - A description used to modify or describe a noun
Enter your amazing code here!
*/
String ageAsString = console.readLine("How old are you? ");
int age = Integer.parseInt(ageAsString);
if (age < 13) {
console.printf("Sorry, you must be at least 13 to use this program. \n");
System.exit(0);
}
String name = console.readLine("Enter your name: ");
String adjective = console.readLine("Enter an adjective: ");
String noun =console.readLine("Enter a noun: ");
if (noun.equalsIgnoreCase("dork") ||
noun.equalsIgnoreCase("jerk"))
{
console.printf("The language is not allowed. Exiting! \n\n");
System.exit(0);
}
String adverb = console.readLine("Enter an adverb: ");
String verb = console.readLine("Enter a verb ending with -ing: ");
console.printf("\nYour TreeStory: \n---------------\n");
console.printf("%s is a %s %s. ", name, adjective, noun);
console.printf("They are always %s %s.\n", adverb, verb);
}
}
It looks like there was some weird formatting with your if statement which the treehouse compiler can be picky about. If this helps, please vote best answer! Happy coding!

Seth Kroger
56,413 PointsLooks like you left off the n in second equalsIg*n*oreCase() on that line.
[also editing question for formatting]

Ryan Carlos
422 PointsThanks both.