Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial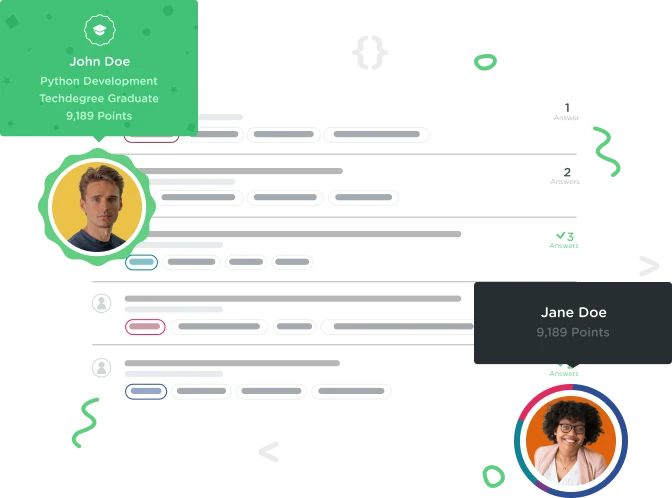

melisa magwizi
1,478 PointsAt which point do I set the attributes for the instance
Kindly advise what is wrong with my code
class RaceCar:
color ="Blue"
fuel_remaining = "50"
def __init__(self,color, fuel_remaining,**kwargs)
self.color = color
self.fuel_remaining = fuel_remaining
for color, fuel_remaining in kwargs.items():
setattr(self,color, fuel_remaining)
1 Answer
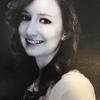
Megan Amendola
Treehouse TeacherHi, Melisa!
class RaceCar:
# these are class attributes
# you don't need these
color ="Blue"
fuel_remaining = "50"
def __init__(self,color, fuel_remaining,**kwargs)
# these are instance attributes
self.color = color
self.fuel_remaining = fuel_remaining
for color, fuel_remaining in kwargs.items():
setattr(self,color, fuel_remaining)
You created 2 class attributes, which you don't need for this challenge. You only need to set 2 instance attributes whose values are passed in when the class is created. You already have the instance attributes set here:
def __init__(self,color, fuel_remaining,**kwargs)
# these are instance attributes
self.color = color
self.fuel_remaining = fuel_remaining
You set instance attributes inside of the __init__
method using self.attribute_name
. For this example, the values will be passed in when an instance of the class is created like this: RaceCar('red', 50)
.
For setattr()
, you can see how Kenneth does it in this video at 3:50. Remember, kwargs
are like dictionary items. They have keys and values. When you create the for loop and set the items, you will need to use key and value instead of color and fuel_remaining. You've already set color and fuel_remaining, kwargs are other items that might be passed. For example: `RaceCar('red', 50, sponsor='Coke Cola', wins=5).
When you remove the class attributes and fix the setattr()
your code should then pass. You've got this!
melisa magwizi
1,478 Pointsmelisa magwizi
1,478 PointsNoted thank you