Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial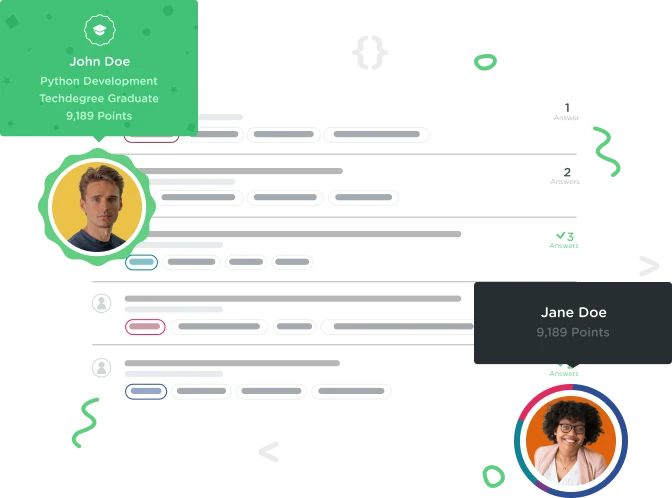
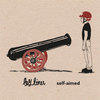
Chris Blues Explosion
4,276 PointsAttempt at Quiz. Suggestions and critics welcome! :)
//Defining variables & constants
/*******************************/
const startButton = document.getElementById('start');
const displayQuestion = document.getElementById('question');
const choice0 = document.getElementById('choice0');
const choice1 = document.getElementById('choice1');
const buttonGuess0 = document.getElementById('guess0');
const buttonGuess1 = document.getElementById('guess1');
const progress = document.getElementById('progress');
const nextQuestion = document.getElementById('next');
const viewScore = document.getElementById('score');
var currentQuestion = 0;
var score = 0;
var fug = new Questions("What was Ian Mackaye's first band?", "Minor Threat", "Black Flag");
var built = new Questions('Which of these is a cult record by "Built to Spill?"', "Keep it like a Secret", "At Action Park");
var myQuiz = new Quiz();
/*******************/
//Defining Function
/*******************/
//Making sure the choices are displayed randomly//
/************************************************/
function random() {
if (Math.floor(Math.random() * 2 < 1)) {
return choice0;
}else {
return choice1;
}
};
/************************/
//Constructor functions//
/************************/
function Questions(question, option1, option2) {
this.question = question;
this.option1 = option1;
this.option2 = option2;
}
function Quiz() {
this.questionsList = [];
}
Quiz.prototype.add = function(question, option1, option2) {
this.questionsList.push(question);
};
myQuiz.add(fug);
myQuiz.add(built);
/*************************/
//Interaction functions//
/***********************/
function removeHtml() {
displayQuestion.innerHTML = "";
choice0.innerHTML = "";
choice1.innerHTML = "";
}
function displayQuestions() {
removeHtml();
displayQuestion.className = "";
displayQuestion.innerHTML = myQuiz.questionsList[currentQuestion].question;
random().innerHTML = myQuiz.questionsList[currentQuestion].option1;
if(choice0.innerHTML === "") {
choice0.innerHTML = myQuiz.questionsList[currentQuestion].option2;
}else{
choice1.innerHTML = myQuiz.questionsList[currentQuestion].option2;
}
progress.innerHTML = "Question " + (currentQuestion + 1) + " of " + myQuiz.questionsList.length;
currentQuestion++;
}
function resetClass() {
choice0.className = "";
choice1.className = "";
}
function hideDisplay() {
choice0.style.display = 'none';
choice1.style.display = 'none';
progress.style.display = 'none';
guess0.style.display = 'none';
guess1.style.display = 'none';
}
function showDisplay() {
choice0.style.display = 'block';
choice1.style.display = 'block';
progress.style.display = 'block';
}
function keepScore() {
if (choice0.className === "selected" && choice0.innerHTML === myQuiz.questionsList[currentQuestion - 1].option1){
score++;
}
if (choice1.className === "selected" && choice1.innerHTML === myQuiz.questionsList[currentQuestion - 1].option1){
score++;
}
}
function displayScore() {
if (score === 0) {
displayQuestion.innerHTML = "You suck...You did not get 1 question right.";
}else if (score === 1){
displayQuestion.innerHTML = "You only got 1 question right.";
} else if (score === 2){
displayQuestion.innerHTML = "Well done!! You got both questions right!";
displayQuestion.className = "winner";
}
}
/**********************************/
//Event Listeners/ DOM Manipulation
/**********************************/
nextQuestion.style.display = 'none';
buttonGuess0.style.display = 'none';
buttonGuess1.style.display = 'none';
viewScore.style.display = 'none';
startButton.addEventListener('click', function() {
score = 0;
displayQuestions();
startButton.style.display = 'none';
nextQuestion.style.display = 'block';
buttonGuess0.style.display = 'block';
buttonGuess1.style.display = 'block';
showDisplay();
});
nextQuestion.addEventListener('click', function() {
keepScore();
resetClass();
displayQuestions();
if (currentQuestion === myQuiz.questionsList.length) {
nextQuestion.style.display = 'none';
viewScore.style.display = 'block';
}
});
buttonGuess0.addEventListener('click', function() {
if (choice1.className = "selected") {
choice1.className = "";
choice0.className = "selected";
}
});
buttonGuess1.addEventListener('click', function() {
if (choice0.className = "selected") {
choice0.className = "";
choice1.className = "selected";
}
});
viewScore.addEventListener('click', function() {
keepScore();
hideDisplay();
displayScore();
viewScore.style.display = 'none';
startButton.innerHTML = "Restart Quiz";
startButton.style.display = 'block';
currentQuestion = 0;
resetClass();
});