Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial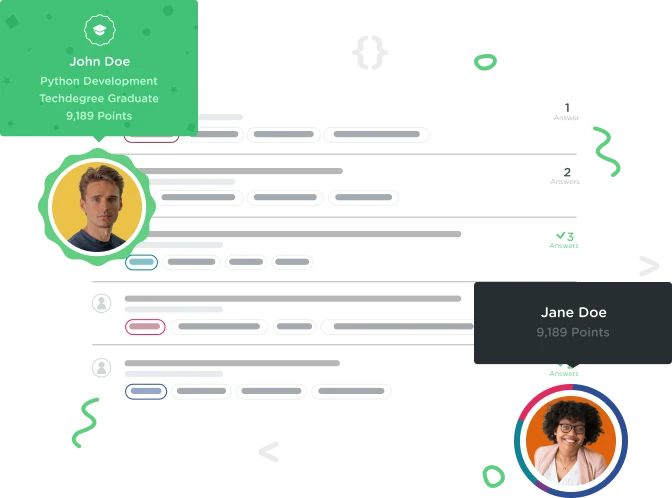

Neil Ganotisi
20,975 PointsAttempting to create an array with a static size, NodeJS won't let me push objects to the array.
When I try to run the following in NodeJS, I end up having a fatal error:
CALL_AND_RETRY_LAST Allocation Failed - process out of memory
Would anyone happen to know why I would have that issue?
// Create a new array with static size of 20 and fill the array with placeholder data.
var array = new Array(20);
for(i=0; i<array.length - 1; i++) {
array.push({name: "=placeholder=",
message: "=placeholder="
});
}
2 Answers
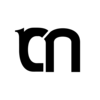
Colin Marshall
32,861 PointsI believe you are seeing this because you have an infinite loop. When you create the array and give it a length of 20 it creates an array with 20 undefined values inside of it. It is not a maximum number of values that can ever be in the array, it's just the number of values (undefined) inside of it when you create it.
Do something like this instead:
var array = new Array();
var arrayMaxLength = 20;
for(i = 0; i < arrayMaxLength; i++) {
array.push({name: "=placeholder=",
message: "=placeholder="
});
}

Seth Kroger
56,413 PointsYou see when you push an object onto the array it's added as an extra element to the end and you're lengthening the array. array.length gets larger every time through the loop and the counter variable i can't catch up, so the loop keeps adding to the array until all the available memory is consumed.
Arrays aren't statically sized in JavaScript anyway so there is no need to use new Array(n). Just push 20 objects onto an empty array.
Neil Ganotisi
20,975 PointsNeil Ganotisi
20,975 PointsProgress has been made and has been resolved, although it isn't as 'clean' as I hoped.
Today I learned, you can create a new array with a static size, but pushing data to the array dynamically expands the array.
Is this the best possible solution? Is there a better method of accomplishing this?