Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial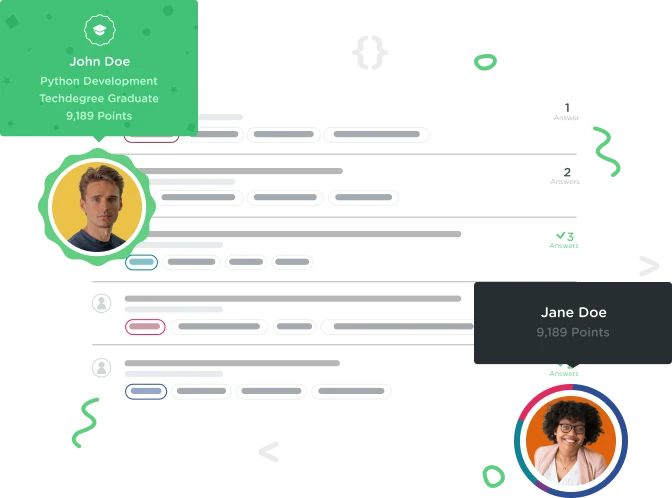
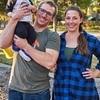
Dehn Hunsworth
7,189 PointsAttempting to practice what i just started learning about the DOM, having trouble getting a simple calculator to functio
I am trying to create a simple ABV calculator for my home brewing. I have tried this a few different ways creating different functions(one of which is commented out here). Each time i get the output to the page but the return is always 0 %. Any suggestion to where I am going wrong is much appreciated!
let ogInput = document.getElementById("ogInput").value; let fgInput = document.getElementById("fgInput").value; const calcSubmit = document.getElementById("calcSubmit"); const abvNum = 131.25; let calcResult = 0.0; let calcReport;
const calcFunc = (num1, num2) => {
calcResult = (num1 - num2) * abvNum;
calcReport = <h2>Your beer is ${calcResult} % ABV</h2>
;
document.getElementById("calcOutput").innerHTML = calcReport;
return false;
};
/*
const displayResult = () => {
calcFunc(ogInput, fgInput);
document.getElementById("calcOutput").innerHTML = calcReport;
return false;
};
*/
calcSubmit.addEventListener("click", calcFunc(ogInput, fgInput));
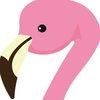
Dave StSomeWhere
19,870 PointsCheck the markdown cheatsheet below to format your code, just ask if you need assistance.
Also, please include your HTML and CSS, if you'd like debugging assistance.
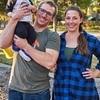
Dehn Hunsworth
7,189 Pointslet ogInput = document.getElementById("ogInput").value;
let fgInput = document.getElementById("fgInput").value;
const calcSubmit = document.getElementById("calcSubmit");
const abvNum = 131.25;
let calcResult = 0.0;
let calcReport;
const calcFunc = (num1, num2) => {
calcResult = (num1 - num2) * abvNum;
calcReport = `<h2>Your beer is ${calcResult} % ABV</h2>`;
document.getElementById("calcOutput").innerHTML = calcReport;
return false;
};
/*
const displayResult = () => {
calcFunc(ogInput, fgInput);
document.getElementById("calcOutput").innerHTML = calcReport;
return false;
};
*/
calcSubmit.addEventListener("click", calcFunc(ogInput, fgInput));
<div class="container">
<section class="calc-container">
<h2>ABV Calculator</h2>
<form action="">
<div class="form-group">
<label for="">Original Gravity: </label>
<input type="text" id="ogInput" />
</div>
<div class="form-group">
<label for="">Final Gravity: </label>
<input type="text" id="fgInput" />
</div>
<button
class="btn btn-primary"
onclick="return displayResult()"
id="calcSubmit"
>
Calculate ABV
</button>
</form>
<div id="calcOutput"></div>
</section>
</div>
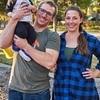
Dehn Hunsworth
7,189 PointsGot it! Thank you much for the quick responses!
1 Answer

Emmanuel C
10,636 PointsHey Dehn,
So I've noticed several things. First, the calcReport which holds the h2 tags youre going to insert should be strings and instead of the curly braces to show the calcResult value, you can concatenate them.
calcReport = '<h2>Your beer is '+ calcResult +'% ABV</h2>';
Second, the addEventListener expects the second parameter to be a call back function, or a function that gets called when the event is trigger, which oddly enough isnt suppose to be the calcFunc youre trying to call. instead calcFunc goes inside the call back function. If not, calcFunc will get called immediately and not when you click the button.
Third, I havent seen your html code, where og and fg Inputs are, But i think your value will be end up as 0 since they only get set once when the script is called. If you enter in something in the input after the script is called, ogInput and fgInput wont update so thier values will stay blank. You can move them into the call back function. So i edited your code and made it look like this.
const calcSubmit = document.getElementById("calcSubmit");
const abvNum = 131.25;
let calcResult = 0.0;
let calcReport;
const calcFunc = (num1, num2) => {
calcResult = (num1 - num2) * abvNum;
calcReport = '<h2>Your beer is '+ calcResult +'% ABV</h2>';
document.getElementById("calcOutput").innerHTML = calcReport;
return false;
};
/* const displayResult = () => {
calcFunc(ogInput, fgInput);
document.getElementById("calcOutput").innerHTML = calcReport;
return false;
};
*/
calcSubmit.addEventListener("click", () => {
let ogInput = document.getElementById("ogInput").value;
let fgInput = document.getElementById("fgInput").value;
calcFunc(ogInput, fgInput);
});
Hope this helps.
Now I seen youre html code, you can remove the onclick attribute from the button tag since youre already adding an event listener in the js, and youll need to add the type attribute to the button of value button to prevent the page from refreshing
<button class="btn btn-primary" id="calcSubmit" type='button'> Calculate ABV </button>
Another way you can do it is by removing the addeventlistener and have the onclick call the calcFunc and have it pass in the ogInput and fgInput. yyoull need to add name attributes to those inputs elements.
<input type="text" id="ogInput" name='og' />
<input type="text" id="fgInput" name='fg' />
<button class="btn btn-primary" id="calcSubmit" type='button' onclick='calcFunc(og.value,fg.value)'>
Calculate ABV
</button>
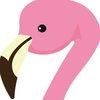
Dave StSomeWhere
19,870 PointsThese seem like good ways to investigate. Here's a Pen with the first way for your coding experimentation.
Dehn Hunsworth
7,189 PointsDehn Hunsworth
7,189 Pointsand that code now looks terrible and hard to read in the post, there a better way to link code in?