Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial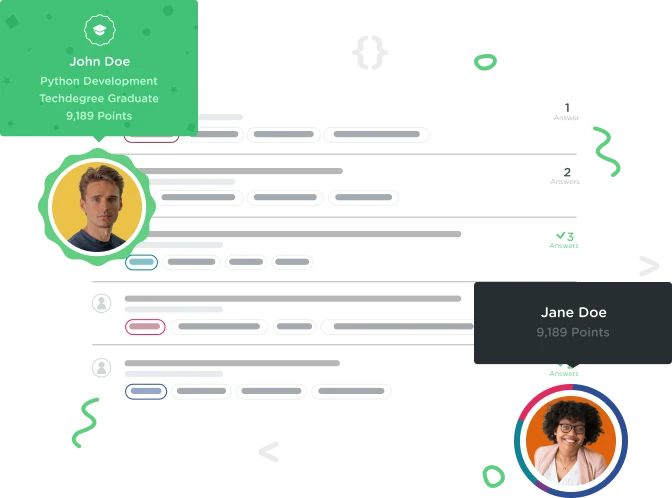

John Locken
16,540 PointsAttempting to streamline final task in Callback Functions in JavaScript
I'm looking to streamline the code to the final task in the Callback Functions course. I understand the easy way to do it, which is to create an addEventListener for each button. I am looking to see if there is a way to do it with a single Event Listener. I tried adding the class "button" to all buttons, then creating a const = event => getElementByClass , but I am getting an error which I will paste below. Is this possible, and if so, what am I missing?
const btn1 = document.getElementById("button1");
const btn2 = document.getElementById("button2");
const btn3 = document.getElementById("button3");
const butClick = event => {event.target.className = 'button'}
function spinElement(event) {
//Applies spinning animation to button element
event.target.className = "spin";
}
butClick.addEventListener('click', spinElement)
<!DOCTYPE html>
<html lang="en">
<head>
<title></title>
<link rel='stylesheet' href='styles.css'>
</head>
<body>
<section >
<button class = 'button' id='button1'>Button 1</button>
<button class = 'button' id='button2'>Button 2</button>
<button class = 'button' id='button3'>Button 3</button>
</section>
<script src='app.js'></script>
</body>
</html>

Cheo R
37,150 PointsI think because you're using a fat arrow function, addEventLIstener uses the this referring to window. So essentially, you're trying to attach addEventListener to window, not butClick.
1 Answer
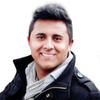
Camilo Lucero
27,692 PointsCreate the event listener over the parent of the buttons and make a condition so the function only executes if the clicked element (event.target) is a button:
const buttonsContainer = document.getElementById("buttons");
function spinElement(event) {
if (event.target.tagName === "BUTTON") {
event.target.className = "spin";
}
}
buttonsContainer.addEventListener("click", spinElement);
<!DOCTYPE html>
<html lang="en">
<head>
<title></title>
<link rel='stylesheet' href='styles.css'>
</head>
<body>
<section id='buttons'>
<button id='button1'>Button 1</button>
<button id='button2'>Button 2</button>
<button id='button3'>Button 3</button>
</section>
<script src='app.js'></script>
</body>
</html>
Even though this works for your goal (preview the HTML to test it), the challenge will show you an error when submitting this answer. Sometimes challenges are designed to check for a specific answer, and don't expect you to get creative :)
John Locken
16,540 PointsJohn Locken
16,540 PointsBummer: There was an error with your code: TypeError: 'undefined' is not a function (evaluating 'butClick.addEventListener('click', spinElement)')