Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial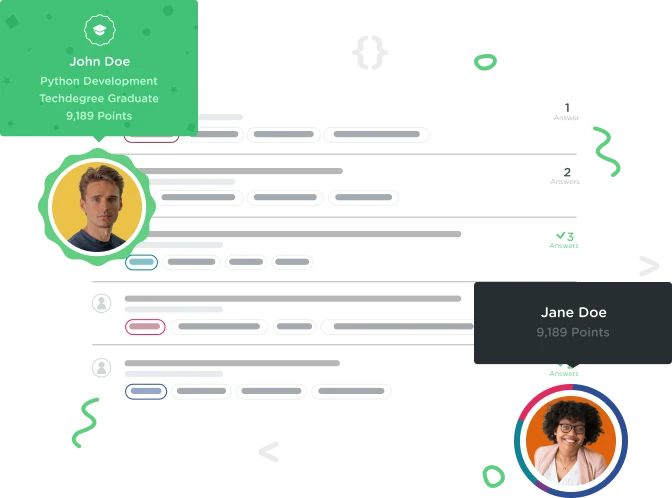

Shreya Tyagi
3,011 PointsAttributeError: 'Thief' object has no attribute 'agile'
class Character:
def __init__(self, name, **kwargs):
self.name = name
for key, value in kwargs.items():
setattr(self, key, value)
import random
class Sneaky:
sneaky = True
def __init__(self, sneaky=True, *args, **kwargs):
super().__init__(*args, **kwargs)
self.sneaky = sneaky
def hide(self, light_level):
return self.sneaky and light_level < 10
class Agile:
agile = True
def __init__(self, agile=True, *args, **kwargs):
super().__init__(*args, **kwargs)
self.agile = agile
def evade(self):
return self.agile and random.randint(0, 1)
import random
from attributes import Agile, Sneaky
from characters import Character
class Thief(Character, Agile, Sneaky):
def pickpocket(self):
return self.sneaky and bool(random.randint(0, 1))
from thieves import Thief
ken = Thief("ken",sneaky = False)
print(ken.sneaky)
print(ken.agile)
print(ken.hide(8))
False
Traceback (most recent call last):
File "play.py", line 4, in <module>
print(ken.agile)
AttributeError: 'Thief' object has no attribute 'agile'
1 Answer
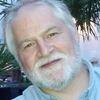
Jeff Muday
Treehouse Moderator 28,726 PointsThis is a good question to ask-- you are discovering an important Python convention!
Python expects the "case" of the import
modules to match the case of the source file. PEP-8 (one of our guiding conventions) there is a suggestion that all filenames should be lowercase.
https://www.python.org/dev/peps/pep-0008/#package-and-module-names
quoting this--
Modules should have short, all-lowercase names. Underscores can be used in the module name if it improves readability. Python packages should also have short, all-lowercase names, although the use of underscores is discouraged.
In your example, to make it work, you should do the following renames.
Characters.py
should be characters.py
Attributes.py
should be attributes.py
Thieves.py
should be thieves.py
Good luck with your Python journey-- Kenneth is a great teacher and has lots of knowledge about multiple resolution order in objects.