Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial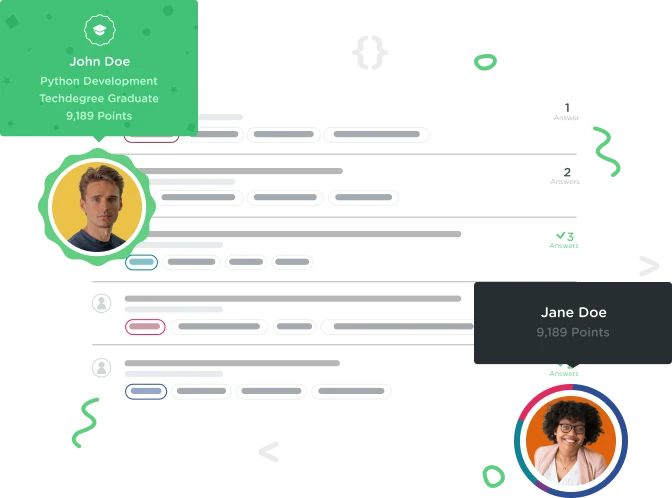
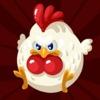
Binyamin Friedman
14,615 PointsAudioClip is abstract; cannot be instantiated.
Here's Home.java I put some spacing around the part that gets an error:
package com.teamtreehouse.pomodoro.controllers;
import com.teamtreehouse.pomodoro.model.Attempt; import com.teamtreehouse.pomodoro.model.AttemptKind; import javafx.animation.Animation; import javafx.animation.KeyFrame; import javafx.animation.Timeline; import javafx.beans.property.SimpleStringProperty; import javafx.beans.property.StringProperty; import javafx.event.ActionEvent; import javafx.fxml.FXML; import javafx.scene.control.Label; import javafx.scene.control.TextArea; import javafx.scene.control.TextField; import javafx.scene.layout.VBox; import javafx.util.Duration;
import java.applet.AudioClip;
public class Home { private final AudioClip mApplause; @FXML private VBox container;
@FXML
private Label title;
@FXML
private TextArea message;
private Attempt mCurrentAttempt;
private StringProperty mTimerText;
private Timeline mTimeline;
public Home() {
mTimerText = new SimpleStringProperty();
setTimerText(0);
mApplause = new
AudioClip(getClass().getResource("/sounds/applause.mp3").toExternalForm());
}
public String getTimerText() {
return mTimerText.get();
}
public StringProperty timerTextProperty() {
return mTimerText;
}
public void setTimerText(String timerText) {
mTimerText.set(timerText);
}
public void setTimerText(int remainingSeconds) {
int minutes = remainingSeconds / 60;
int seconds = remainingSeconds % 60;
setTimerText(String.format("%02d:%02d", minutes, seconds));
}
private void prepareAttempt(AttemptKind kind) {
reset();
mCurrentAttempt = new Attempt("", kind);
addAttemptStyle(kind);
title.setText(kind.getDisplayName());
setTimerText(mCurrentAttempt.getRemainingSeconds());
mTimeline = new Timeline();
mTimeline.setCycleCount(kind.getTotalSeconds());
mTimeline.getKeyFrames().add(new KeyFrame(Duration.seconds(1), e -> {
mCurrentAttempt.tick();
setTimerText(mCurrentAttempt.getRemainingSeconds());
}));
mTimeline.setOnFinished(e -> {
saveCurrentAttempt();
prepareAttempt(mCurrentAttempt.getKind() == AttemptKind.FOCUS ?
AttemptKind.BREAK : AttemptKind.FOCUS);
mApplause.play();
});
}
private void saveCurrentAttempt() {
mCurrentAttempt.setMessage(message.getText());
mCurrentAttempt.save();
}
private void reset() {
clearAttemptStyles();
if(mTimeline != null && mTimeline.getStatus() == Animation.Status.RUNNING) {
mTimeline.stop();
}
}
public void playTimer() {
mTimeline.play();
}
public void pauseTimer() {
mTimeline.stop();
}
private void addAttemptStyle(AttemptKind kind) {
container.getStyleClass().add(kind.toString().toLowerCase());
}
private void clearAttemptStyles() {
for(AttemptKind kind : AttemptKind.values()) {
container.getStyleClass().remove(kind.toString().toLowerCase());
}
}
public void DEBUG(ActionEvent actionEvent) {
System.out.println("Hi!");
}
public void handleRestart(ActionEvent actionEvent) {
prepareAttempt(AttemptKind.FOCUS);
playTimer();
}
}
1 Answer
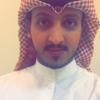
Fahad Mutair
10,359 Pointshi Binyamin Friedman , you have imported the wrong AudioClip so remove this line import java.applet.AudioClip;
it should be
import javafx.scene.media.AudioClip;
Fahad Mutair
10,359 PointsFahad Mutair
10,359 Pointsuse markdown cheatsheet for the code, it will be easy to read