Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial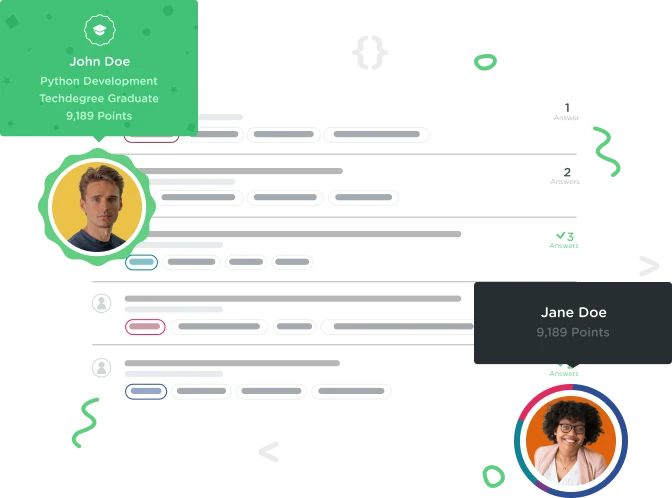
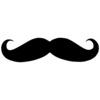
hum4n01d
25,493 PointsAuto Fullscreen Videos
Hi everyone, I am making a website with a video and I want it to automatically go into fullscreen and play when clicked. I already have the play on click script but I don’t know how to make it go into fullscreen.
These might be helpful:
https://developer.mozilla.org/en-US/docs/Web/Guide/API/DOM/Using_full_screen_mode
http://robnyman.github.io/fullscreen/
Thanks!
2 Answers
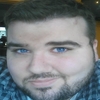
Marcus Parsons
15,719 PointsHey 64yes,
I did find a way to make the video go full screen when you click play, but it has to be done through a button. It cannot be done by clicking play on the video. What I did was not display the controls for the webm and then add a button that plays/pauses the video. When you hit play, it automatically jumps into full screen mode. You'd want to add some other buttons too for functionality, but this is just a very basic example.
You'll need to change the source for the video (and the type of video) to whatever it is you'd like to play. Mess around with it!
Here is the simple page:
<!DOCTYPE html>
<html>
<head>
<title>HTML5 Video</title>
</head>
<body>
<video id="myVideo" width="400" height="400">
<source src="Videos/cod.webm" type="video/webm">
</video>
<button id="playButton">Play/Pause</button>
<script type="text/javascript">
var myVideo = document.getElementById('myVideo');
var playButton = document.getElementById('playButton');
playButton.addEventListener('click', function () {
if (myVideo.paused) {
if (myVideo.requestFullscreen) {
myVideo.requestFullscreen();
}
else if (myVideo.msRequestFullscreen) {
myVideo.msRequestFullscreen();
}
else if (myVideo.mozRequestFullScreen) {
myVideo.mozRequestFullScreen();
}
else if (myVideo.webkitRequestFullScreen) {
myVideo.webkitRequestFullScreen();
}
myVideo.play();
}
else {
myVideo.pause();
}
}, false);
</script>
</body>
</html>
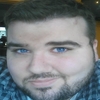
Marcus Parsons
15,719 PointsHi 64yes,
You can't force a page to automatically enter in Fullscreen mode, at least in Webkit based browsers. You have to make a request to enter full screen so that it can be toggled by the user. In Windows' Internet Explorer, you can do some sneaky "window.open" tricks, but I wouldn't advise you to use them. It would be frustrating to go to a website and have a video/page automatically load in full screen when I didn't tell it to.
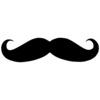
hum4n01d
25,493 PointsThen how does this work? http://robnyman.github.io/fullscreen/
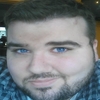
Marcus Parsons
15,719 PointsApologies. I misread your question. I thought you wanted the video to automatically play and go into full screen mode when the page was done loading.
hum4n01d
25,493 Pointshum4n01d
25,493 PointsThank you!
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsYou are very welcome :)