Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial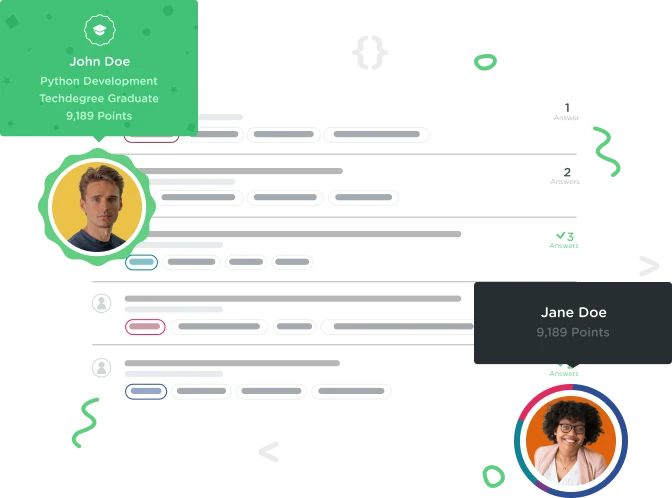

Michael Wiedenheft
229 PointsAuto Incrementing at the end?
Curious as to why the auto incrementing has to be done at the end. Could someone elaborate please?

Michael Wiedenheft
229 PointsYes Jason, around the 2:26 mark where he states that is it important to place the auto-incrementing last. Why do you get a different return when the '++w' is placed before the printf statement when compared to placing it after the printf statement?
1 Answer

Jason Anello
Courses Plus Student 94,610 PointsIt's not so much that it has to be done at the end but I think it's more intuitive to do it there.
You can increment at the beginning of the loop but you have to make some adjustments.
Here's some code you can try out:
#include <stdio.h>
int main()
{
char letters[] = { 'a', 'b', 'c' };
int w =0;
while (w < 3) {
printf("letter %d is %c\n", w, letters[w]);
++w;
}
printf ("\n\n");
//This produces the same output
int i = -1;
while (i < 2) {
++i;
printf("letter %d is %c\n", i, letters[i]);
}
return 0;
}
To make it work you have to lower the starting value by 1 and also the loop condition.
In the 1st loop it seems more intuitive to start at the 1st value you need (0) and loop as long as the index is less than the length of the array (3). This is a common pattern in programming when working with arrays.
In the second one, you have to make adjustments to account for incrementing right away. The problem with starting at 0 is that you increment it right away and it becomes 1. You never get a chance to use the 0 to access the 'a' element in the array. You have to start at -1 so that it becomes 0 when incremented right away.
Let me know if you need any further clarification.

Michael Wiedenheft
229 PointsI believe that I understand. I think I was unaware that printf was doing more than displaying the values, but outputting results to be interpreted. Thank you Jason.

Jason Anello
Courses Plus Student 94,610 Pointsprintf
is only displaying the values. Could you clarify what you mean by "outputting results to be interpreted"?
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsHi Michael,
Are you talking about the
while
loop around the 2:00 mark?