Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial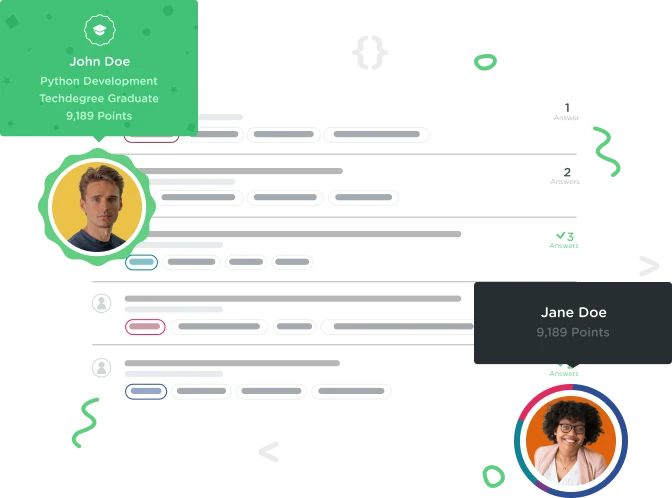
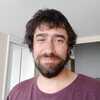
Rodrigo Muñoz
Courses Plus Student 20,171 PointsAutomatically updating state when expiration has come
I have a field of a model that changes states but I need to automatically modify that value after an expiration datetime has come. Is there a way to do this?
Here is an example:
class Post(models.Model):
created_at = models.DateTimeField(auto_now_add=True)
state = (
('1', 'active'),
('2', 'inactive'),
)
state = models.CharField(max_length=20, choices=state, default='active')
duration = models.DurationField(blank=True, null=True)
expires = models.DateTimeField(blank=True, null=True)
updated_at = models.DateTimeField(auto_now=True)
@property
def active(self):
return self.expires > localtime(now())
def save(self, *args, **kwargs):
self.created_at = localtime(now())
self.expires = self.created_at + self.duration
return super(Post, self).save(*args, **kwargs)
The problem I have is that I have to call the active function property to check if it's True or False and I'm looking for a way that this can be done automatically so the database updates itself. I've been thinking about Django-cron or Celery but I can't chose when to call the active function, this should be done for itself.
Is this possible?
1 Answer
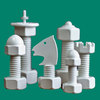
Steven Parker
229,783 PointsScheduled operations in a database are a pain and create opportunities for failures. They are best used when no other solution can accomplish the goal.
For what you are doing, I would think your existing strategy is already superior. Why would calling your property be more trouble than just examining the state value?
Rodrigo Muñoz
Courses Plus Student 20,171 PointsRodrigo Muñoz
Courses Plus Student 20,171 PointsThe problem I have is that I'm looking for a way to change the state based on expiration, without the need of calling the property. I have added this:
But it doesn't work neither.