Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial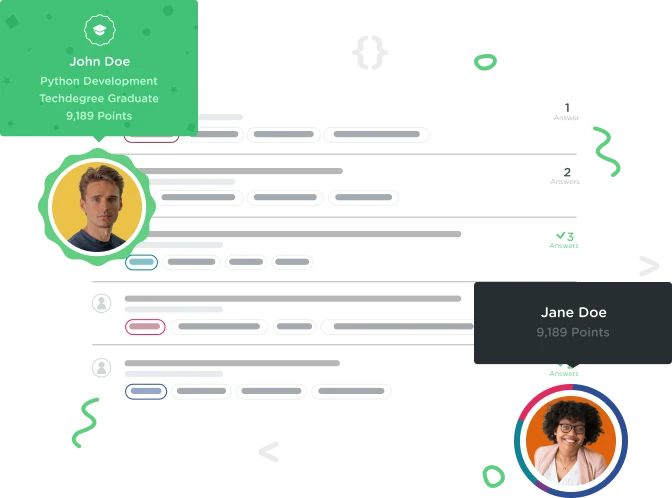

RODRIGO MARTINEZ
5,912 PointsAverage from array using reduce. Add your own method to the Array object so the following code would work. 3 approaches.
I'm trying to solve a task.
The following code should work adding my own method. It's a beginner excercise for getting an average. I could have done it with a for loop maybe, but i was trying to implement the reduce method.
This is the given code
var arr = [1, 2, 3, 4, 5];
var avg = arr.average();
console.log(avg);
I've been able to get the average with the following code.
var arr = [1, 2, 3, 4, 5];
function average() {
const accPlusCur =(acc,cur) => acc + cur;
const sumOfAll = arr.reduce(accPlusCur);
return sumOfAll / arr.length;
};
console.log(average());
// => 3
So i re wrote it this other way to try to have a function that i could apply to any array and not just this specific one. This one also works.
const arr = [1, 2, 3, 4, 5];
function average(x) {
const sumOfAll = x.reduce((acc, cur) => acc + cur, 0);
return sumOfAll / x.length;
};
const averageVariable = average(arr);
console.log(averageVariable);
The solution given online is the following. Although i don't really quite get it.
Array.prototype.average = function() {
var sum = this.reduce(function(prev, cur) { return prev + cur; });
return sum / this.length;
}
var arr = [1, 2, 3, 4, 5];
var avg = arr.average();
console.log(avg); // => 3
Why is Array word before prototype? Is it like declaring a function called "average"and then i call it in the "avg" variable?
2 Answers

anthony amaro
8,686 Pointsyour solution works fine. and what do you mean by The problem is i'm not really using exactly the given code and you may want to use Math.floor to get a whole number. if you use an array with more items in it. you are probably going to get a weird number like 3.234544322
return Math.floor(sumOfAll / x.length);

anthony amaro
8,686 Pointstry checking this out https://javascript.info/native-prototypes
RODRIGO MARTINEZ
5,912 PointsRODRIGO MARTINEZ
5,912 PointsThanks Antonhy for response. I had not corrected/edited the post. I'll add the math .floor method. It's just not very clear to me the solution they gave me for that problem. The last one with Array.prototype