Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial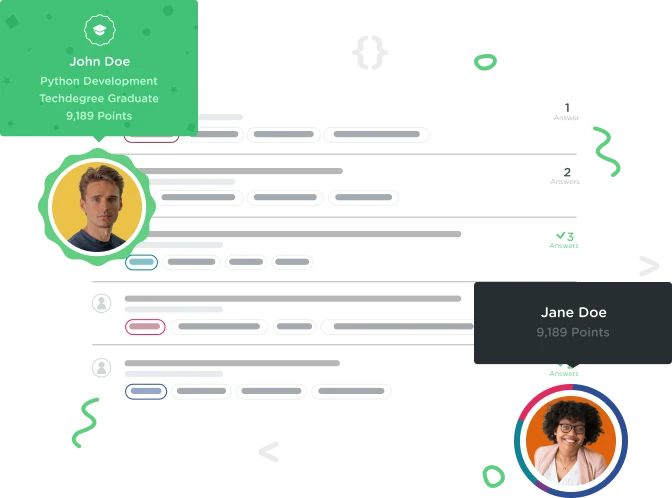

Dominic Ratcliff
9,425 PointsAverager challenge: did you do?
Below is my code, anyone else care to share how they coded this?
using System;
namespace Treehouse.Averager
{
class Averager
{
static void Main()
{
var runningTotal = 0.0;
var count = 0.0;
while(true)
{
//Prompt user for number or done
Console.Write("Enter a number or type \"done\" to see the average: ");
string newNum = Console.ReadLine();
if (newNum.ToLower() == "done")
{
break;
}
try
{
double toAdd = double.Parse(newNum);
runningTotal += toAdd;
count += 1;
}
catch (FormatException)
{
Console.WriteLine("That is not valid input.");
}
}
Console.WriteLine("Average Rounded to 2 Places: " + Math.Round((runningTotal/count), 2));
}
}
}
Alistair Mackay
7,812 PointsAlistair Mackay
7,812 PointsI like the rounding to two decimal places, that's a nice touch.
I struggled through and came up with something like this, the total value isn't required but I liked the way it turned out in the end:
my main problem was my tryCount variable would increment even if what the user input was the wrong type. This would mean my average value would be wrong going forward.
double userValue = double.Parse(userInput);
Parsing the input solved the problem as any input that wasn't a number after that point would throw a format exception before hitting the increment.
Good to see I was on the right path. Thanks for the share!
-PC