Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial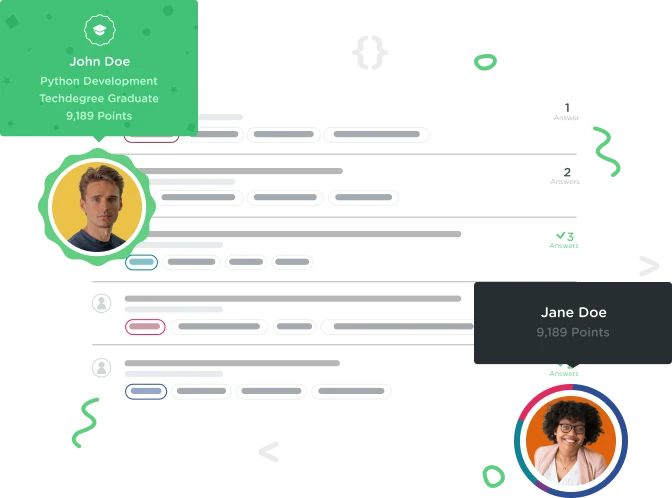

Richard Pitts
Courses Plus Student 1,243 PointsAye, I thought I got it right, please help!
Aye, I thought I got it right, please help!
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
enum Direction {
case left
case right
case up
case down
}
class Robot {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: .left) {
// Enter your code below
-> Point(x: x-1, y: y)
}
}
1 Answer
Dan Lindsay
39,611 PointsHello Richard,
Yeah, this was a tricky one for me to figure out too. There are two ways(that I know of) that we can do this. First, we donβt want to change the function parameter type, we want to keep it as Direction. Only when calling the function would we use .left(or any of the other three cases of the Direction enum). Second, we want to switch on the internal variable(direction), which is of type Direction, and make our changes to location there, like so:
func move(_ direction: Direction) {
// Enter your code below
switch direction {
case .left:
location = Point(x: -1, y: 0)
case .right:
location = Point(x: 1, y: 0)
case .up:
location = Point(x: 0, y: 1)
case .down:
location = Point(x: 0, y: -1)
}
}
Of course we could say case Direction.left(and so on for the other cases), but we know that direction is of type Direction, so we can omit it. The shorter way to do it is just referencing the x and y properties of our location property and add or subtract 1, depending on the case, like so:
func move(_ direction: Direction) {
// Enter your code below
switch direction {
case .left:
location.x -= 1
case .right:
location.x += 1
case .up:
location.y += 1
case .down:
location.y -= 1
}
}
Both of those should work. I find these challenges a lot easier to figure out when doing them in an Xcode Playground first. Good olβ code completion helps so much!
I hope I explained it well enough for you, and that it helps make it a bit easier to understand.
Dan