Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial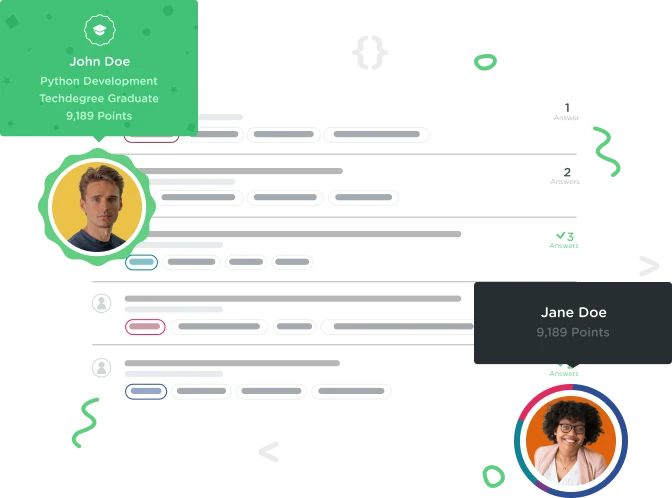

Robert McElvenny
3,943 Pointsbackground color is not updating properly for the first image nor is it updating to the UIImageView, code is identical.
All the code looks the same from start to finish, everything else works but for some reason the backgroundColor isn't updating properly from the colorDictionary

X. Szuh
2,486 PointsLook in rgbColorFromDictionary method. You have misspelled alpha.
2 Answers

X. Szuh
2,486 PointsILook in rgbColorFromDictionary method. You have misspelled alpha.

Robert McElvenny
3,943 PointsI can't believe just that slight typo broke it. :P Ty again!

Robert McElvenny
3,943 PointsI can't believe just that slight typo broke it. :P Ty again!

Damien Watson
27,419 PointsHey Robert, A bit hard to tell without being able to debug myself, but a quick thing you might be able to check:
PlaylistDetailViewController -> Instead of setting background color to 'self.playlist.backgroundColor' try setting it to [UIColor redColor]. This will identify if the background is being set properly.
If that works, check the color is successfully being created and passed in.
Hope that helps some.

Damien Watson
27,419 PointsOoops, I didn't mean to put this in as an answer... I think Deanne is on the money.

Robert McElvenny
3,943 PointsThank you though!
Robert McElvenny
3,943 PointsRobert McElvenny
3,943 Points