Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial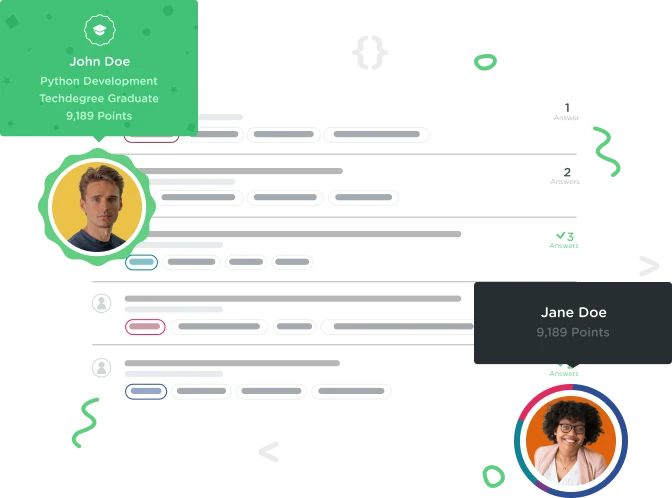

yahya hussein
2,516 PointsBackwards Logic?
I think my logic is backwards now. For some reason whenever I guess a correct letter, the number of chances I have to solve the word go down instead of when I enter an incorrect letter. Also my '-''s aren't being replaced by the letter anymore. I'm confused as to why this is happening, I've followed along line by line but still getting this bug. No errors are being thrown. Any help will be appreciated.
Game.java file
class Game {
public static final int MAX_MISSES = 7;
private String answer;
private String hits;
private String misses;
private char normalizeGuess(char letter){
// Check if user input is not a letter
if (! Character.isLetter(letter)) {
throw new IllegalArgumentException("A letter is required");
}
letter = Character.toLowerCase(letter);
// Apply check to see if letter has already been guessed
if (misses.indexOf(letter) != -1 || hits.indexOf(letter) != -1) {
throw new IllegalArgumentException(letter + " has already been guessed");
}
return letter;
}
// Constructor for answer
public Game(String answer) {
this.answer = answer.toLowerCase();
hits = "";
misses = "";
}
// Method to apply guess towards answer
public boolean applyGuess(char letter){
letter = normalizeGuess(letter);
boolean isHit = answer.indexOf(letter) != -1;
if (!isHit) {
hits += letter;
}else {
misses += letter;
}
return isHit;
}
public int getRemainingTries(){
return MAX_MISSES - misses.length();
}
// Method that will return a string and show dashes for unguessed letters
public String getCurrentProgress(){
String progress = "";
for (char letter : answer.toCharArray()) {
char display = '_';
if (hits.indexOf(letter) != -1) {
display = letter;
}
progress += display;
}
return progress;
}
}
PRompter.java import java.util.Scanner;
class Prompter {
// Prompt user for input by storing instance of game inside variable
private Game game;
public Prompter(Game game){
this.game = game;
}
public boolean promptForGuess(){
Scanner scanner = new Scanner(System.in);
boolean isHit = false;
// Store new value to keep track of state
boolean isAcceptable = false;
// Loop to keep checking if input is valid
do {
// Prompt user for input
System.out.println("Enter a letter: ");
// Get input
String guessInput = scanner.nextLine();
char guess = guessInput.charAt(0);
try{
// Normalize user input
isHit = game.applyGuess(guess);
// Change value to true
isAcceptable = true;
}catch(IllegalArgumentException iae){
System.out.printf("%s. Please try again. ",
iae.getMessage());
}
}while(! isAcceptable);
return isHit;
}
public void displayProgress(){
System.out.printf("You have %d tries left to solve: %s%n ",
game.getRemainingTries(),
game.getCurrentProgress());
}
}
1 Answer

Zachary Miles
5,377 Points"For some reason whenever I guess a correct letter, the number of chances I have to solve the word go down instead of when I enter an incorrect letter."
This is because in your applyGuess() method in the your Game class you have "if (!isHit)" on line four of the method. So, your code is saying if the variable isHit is not true, then add the letter to the hit String, but what you are supposed to do is add the letter to the hit String if isHit is true. To fix this you need to remove the NOT operator and have it read: if(isHit). This should also resolve the other issue you are having.
Hope this helped you.
Cheers!