Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial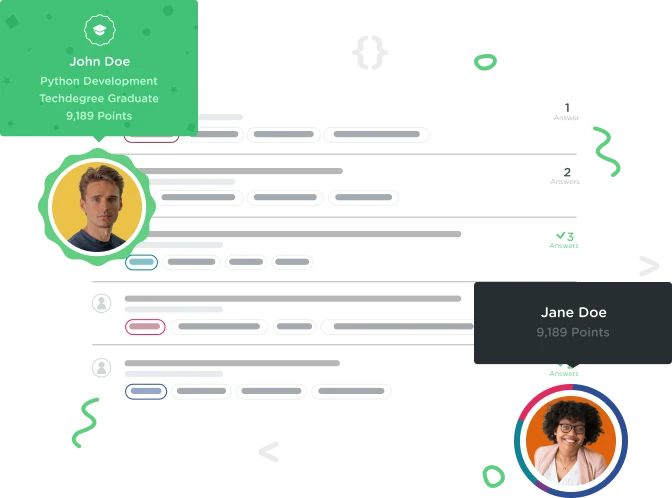

Jaxon Gonzales
3,562 PointsBackwards Number Guessing Game in Python
Hi! I am trying to make a backwards version of the number guessing game in python in which the user inputs a number and the computer guesses what the number is until it guesses correctly. I have run into a problem though. At the end I put a question asking whether or not the user would like to play again. If they say yes the game starts again but does not finish with the usual "The computer guessed your.... in {} guesses" it instead leaves that sentence off and ends the document.
Here is my code:
import random
def game_loop():
high_num = 25
low_num = 1
guess_count = []
#Ask the user for their number
secret_num = int(input("What would you like the secret number to be? (1-25)"))
#Have computer guess a number
while True:
computer_guess = random.randint(low_num, high_num)
#Make sure computer does not guess the same number twice
if computer_guess in guess_count:
computer_guess = random.randint(low_num, high_num)
break
#Tell computer if its number is too high/low
if computer_guess > secret_num:
high_num = computer_guess
guess_count.append(computer_guess)
print("{} is too high!".format(computer_guess))
elif computer_guess < secret_num:
low_num = computer_guess
guess_count.append(computer_guess)
print("{} is too low".format(computer_guess))
else:
guess_count.append(computer_guess)
print("The computer guessed your secret number {} in {} guesses!".format(secret_num, len(guess_count)))
play_again = input("Whould you like to play again? (Y/N) ")
if play_again.lower() == "y":
game_loop()
else:
quit()
#Play again?
game_loop()
Any help is appreciated!?
2 Answers
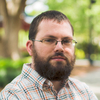
Kenneth Love
Treehouse Guest TeacherI don't see anything in your script that stands out to me as being wrong. You might try changing it to return game_loop()
if they choose to play again instead of just calling game_loop
a second time.
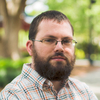
Kenneth Love
Treehouse Guest TeacherComing back to this after awhile. I bet it's hitting that break
in your first if
. What about just putting continue
inside that if
and nothing else? Then if it guesses a number it's already guessed, it'll just start the loop over.
Jaxon Gonzales
3,562 PointsJaxon Gonzales
3,562 PointsThanks for the answer! I did what you suggested but the program still does not function correctly. I will attach a picture below of what is happening.
Thanks again for your help.
https://pasteboard.co/GzA71Sq.png