Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial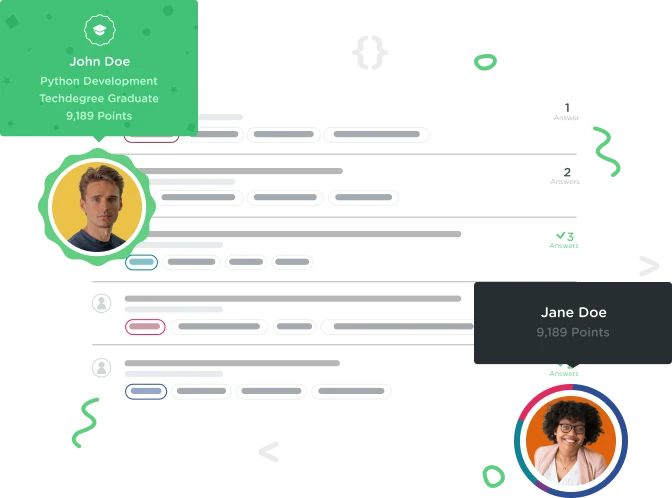

pratyush raj
7,097 Pointsbad operand type
public class Explore {
public static void main(String[] args) { // Create a new friends array using the array literal shortcut. // Include Ben, Alena, Pasan String[] friends = {"Ben", "Alena", "Pasan"}; // Enhanced for loop int[][] scoreCards = { {1, 2, 4, 2, 6, 5, 4, 3, 3, 2, 5, 7, 2, 7, 8, 4, 3, 2}, {2, 3, 5, 1, 1, 2, 3, 1, 1, 2, 4, 1, 3, 3, 2, 6, 3, 2}, {4, 4, 2, 1, 2, 2, 1, 4, 2, 2, 2, 3, 2, 5, 8, 1, 2, 2} }; for(int i=0;i<friends.length;i++) { System.out.printf("%s___________%n",friends[i]); for (int j=0;j < scoreCards[i].length;j++){ System.out.printf("hole #%d : %d %n",j+1,scoreCards[i][j]); } }
}
}
it is saying that in the 2nd for loop i am using a bad operand type
1 Answer
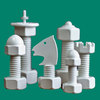
Steven Parker
240,449 PointsWithout formatting, most of the program appears as a single-line comment. But after reconstructing, it complied and ran without errors. Perhaps the original has some issue with the way it's laid out?
Here's the reconstruction shown with Markdown formatting:
public class Explore {
public static void main(String[] args) {
// Create a new friends array using the array literal shortcut.
// Include Ben, Alena, Pasan
String[] friends = {"Ben", "Alena", "Pasan"};
// Enhanced for loop
int[][] scoreCards = { {1, 2, 4, 2, 6, 5, 4, 3, 3, 2, 5, 7, 2, 7, 8, 4, 3, 2},
{2, 3, 5, 1, 1, 2, 3, 1, 1, 2, 4, 1, 3, 3, 2, 6, 3, 2},
{4, 4, 2, 1, 2, 2, 1, 4, 2, 2, 2, 3, 2, 5, 8, 1, 2, 2} };
for(int i=0; i<friends.length; i++) {
System.out.printf("%s___________%n", friends[i]);
for (int j=0; j < scoreCards[i].length; j++) {
System.out.printf("hole #%d : %d %n", j+1, scoreCards[i][j]);
}
}
}
}