Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial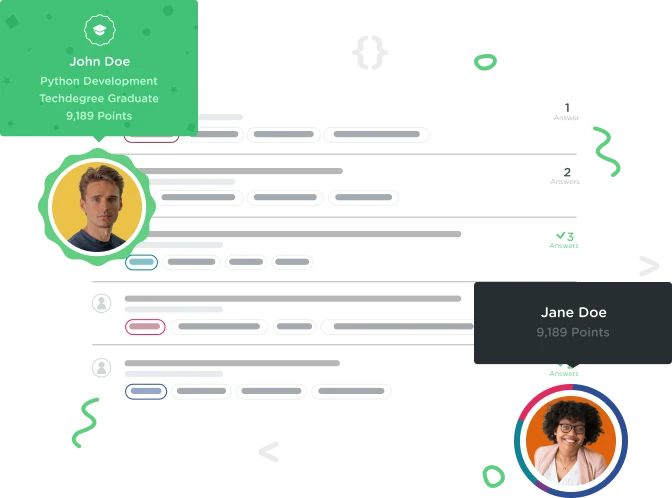
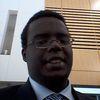
Leonard Morrison
Courses Plus Student 32,914 PointsBase exception doesn't work on this test.
For some Reason, the base exception does not work when I try to test the program. Craig's been talking about it like it's real important and all, but I fail to see why I shouldn't use the specific exception. Does it not cover it? Did the InvalidLocationException suddenly become a special exception? What gives?
package com.teamtreehouse.vending;
import org.junit.Before;
import org.junit.Test;
import static org.junit.Assert.*;
/**
* Created by Leban on 2016-06-09.
*/
public class AlphaNumericChooserTest {
private AlphaNumericChooser chooser;
@Before
public void setUp() throws Exception {
chooser = new AlphaNumericChooser(26, 10);
}
@Test
public void validInputReturnsProperLocation() throws Exception{
AlphaNumericChooser.Location loc = chooser.locationFromInput("D2");
assertEquals("Right row", 3, loc.getRow());
assertEquals("", 1, loc.getColumn());
}
@Test
public void choosingWrongInputIsNotAllowed() throws Exception {
chooser.locationFromInput("WRONG");
}
}
3 Answers
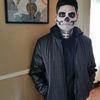
Jorge Otero
16,380 PointsThis error occur cuz in the version of the project "Vending" that you are using InvalidLocationException extends to Throwable, so if you open InvalidLocationException and change Throwable for Exception it will work.
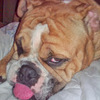
Shane Robinson
7,324 PointsIf I'm not mistaken it should be:
@Test(expected = Exception.class)
Instead of only @Test. Because @Test will consider any exception being thrown as a failure. I don't know if that actually answers your question though. :s

Simon Coates
28,694 PointsGiven the current state of the code, the solution might be to change InvalidLocationException to extend Exception (rather than throwable) or try something like:
@Test (expected = InvalidLocationException.class)
public void choosingWrongInputIsNotAllowed() throws Throwable {
alpha.locationFromInput("B4");
}
Simon Coates
28,694 PointsSimon Coates
28,694 PointsI think the code that Craig Dennis includes currently, the InvalidLocationException isn't an exception, but extends throwable (the superclass of exception). A couple other users have noted they've had trouble with this.