Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial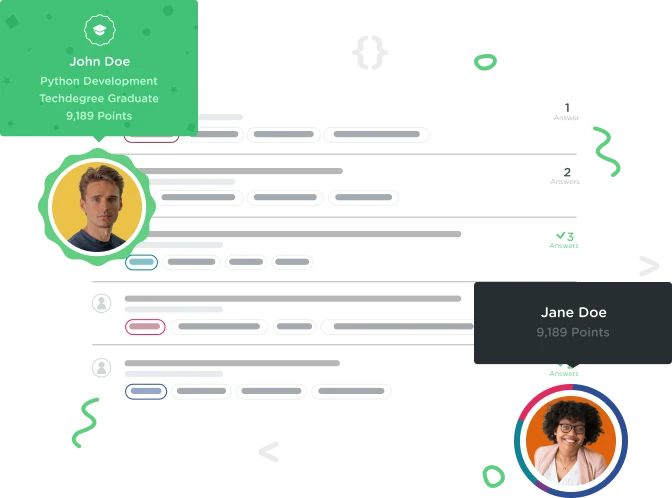

Nyki Lawson
1,820 PointsBasic Ruby Coding
Just finished Basic Ruby. Playing with some basic function/coding/etc. Unable to figure out what is causing/how to stop the errors in my below code. The error is below the code snippet, minus the numerical input.
print "Please enter your name: "
name = gets
puts "Hello #{name}"
print "What is your age? "
age = gets
puts "Thank you. Doing calculations."
results = (age / 2 + 7)
puts "Calculations complete. Results: #{results}"
testing.rb:9:in <main>': undefined method
/' for "(Numerical input for age)\n":String (NoMethodError)
2 Answers

Stone Preston
42,016 Pointsyou assign the age variable the string entered by the user. since gets returns a string you need to convert the return value of gets to an integer to be able to do math on it:
print "Please enter your name: "
name = gets
puts "Hello #{name}"
print "What is your age? "
//convert whatever the user enters to an integer
age = Integer(gets)
puts "Thank you. Doing calculations."
results = (age / 2 + 7)
puts "Calculations complete. Results: #{results}"
this of course assumes the user is going to enter integers each time.
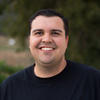
Nicholas Olsen
Front End Web Development Techdegree Student 19,342 PointsI suspect "gets" gives you a string, so you'll have to convert it to a number if you want to do math with it. You can see that ruby strings have a "to_i" method that converts them into integers.
print "What is your age? "
age = gets.to_i
results = (age / 2 + 7)
puts "Calculations complete. Results: #{results}"
There is one other thing you'll have to look out for: if the user provides "0" as their age, you will get an error. You'll have to check and make sure it isn't 0 if you want to avoid that.