Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial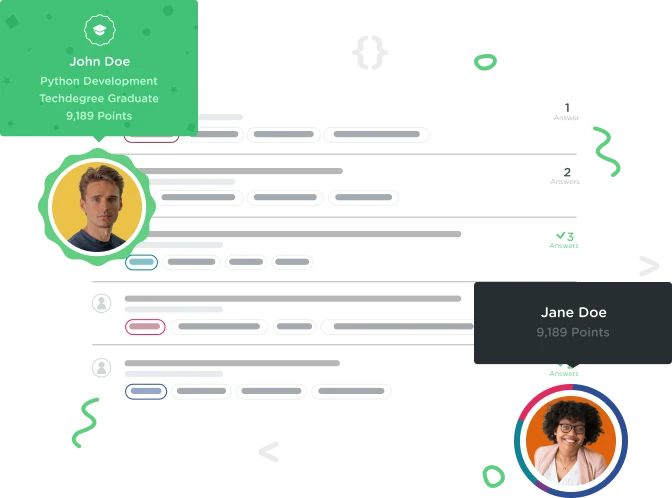

Keyan Abtahi
Python Web Development Techdegree Student 267 PointsBasic VAR question: How do I use a local var in a function without global?
Hi!
I keep having the same problem whenever I write code. I can't seem to figure out how to use the vars outside of a function inside a functions scope.
Google tells me to "You can use global but DONT use global", or only complex examples I dont fully grasp yet.
- If I try to use a var that is outside of a functions scope, I get an error.
- If I move said var to the functions scope and make it local, the function works BUT the rest of the code where that local var is used creates errors.
If I have to use the same vars in different functions, do I have to declare them in every single function? Can I pass vars from one function to another function??
Can someone explain to me like I'm 5? Thank you!
6 Answers

Eric Butler
33,512 PointsI had to look up the syntax -- it looks like you need to structure the "return" syntax a little differently for Python, but I think this is how you'd do it:
def doStuff():
thing1 = "Hello"
thing2 = "Good Bye"
return { "thing1": thing1, "thing2": thing2 }
doStuffVariables = doStuff()
print(doStuffVariables["thing1"])
print(doStuffVariables["thing2"])
print(doStuffVariables)
Cheers, Keyan, and good luck to you!

Eric Butler
33,512 PointsHey Keyan, Your suspicions are right, you don't want to re-declare the same variables over and over in every function. I'll try to keep it simple. If you have variables that are outside of a function, and you want to use them inside the function, the easiest way to do that is to pass them into the function as arguments. That looks like this:
// Here is your global variable:
var whatever = "This variable is outside the function, in the global scope.";
// Here is the function definition, which establishes that the function can take an argument ["arg"]:
function doStuff(arg) {
alert(arg); // This just pops up an alert modal with whatever the value of the argument is.
}
// Now when you actually call (execute) the function, if you pass in the global variable, the function will use it!:
doStuff(whatever); // should alert "This variable is outside the function..."
You can pass in as many arguments as you want:
function doStuff(arg1, arg2, arg3) {
// and now you can do anything you want with any of those passed-in variables:
console.log(arg1);
alert(arg2);
var newThing = parseInt(arg3, 10) * 5;
return arg1 + " " + arg2;
}
doStuff("Hello", "Woohoo!", 7);
And while it's true that it's better not to leave a bunch of stuff in the global scope, it's totally fine for small websites and totally fine when you're still learning the basics. You don't need to over-optimize when you're still learning the basic concepts.
Hope that helps you! Let me know if it's still not making sense.

Eric Butler
33,512 PointsWhoops, I had JavaScript on the brain, but I just saw you flagged this as a Python question. Luckily, the exact same concept holds true, you just need new syntax:
whatever = "This variable is outside the function"
def doStuff(arg):
print(arg)
doStuff(whatever)
Make sense?

Eric Butler
33,512 PointsThe only way to get things out of a function is to "return" them, and a function can only return 1 single "thing" - that's how functions work. So, if you want to return several variables/values out of a function to use elsewhere, you have to collect them into an object first, and then return that object. Then you have to assign that returned object as the value of a new variable that's in the global scope.
It's probably easier to understand in an example (I'm using JavaScript again since I know that syntax better - again, the concept is the same in Python and other languages):
function doStuff() {
// Here are some variables inside a function:
var thing1 = "Hello";
var thing2 = "Good Bye";
// To get them out of the function, create an object (using curly braces) with the variables in it, and have that be what the function returns:
return {
thing1,
thing2
}
}
// Now call (execute) the function to get the variables out to a new variable (doStuffVariables) in the global scope:
var doStuffVariables = doStuff();
// Now you can use them! doStuffVariables is an object and thing1/thing2 are properties, with "Hello" and "Good Bye" as their values.
console.log( doStuffVariables.thing1 ); // logs "Hello"
console.log( doStuffVariables.thing2 ); // logs "Good Bye"
// And if you want to see all your variables and their values (for debugging etc):
console.log( doStuffVariables );
Hope all that makes sense again!

Keyan Abtahi
Python Web Development Techdegree Student 267 PointsHi again Eric! The way you explain things are very helpful, I do understand the concept behind what you just taught me.
The issue is the error I get: AttributeError 'set' object has no attribute 'bet'
The code I use is: https://pastebin.com/vMEmB7ZP
Your code works in JS though, perfectly and exactly like I want it to, so it has to be Python related..
Thank you once again for your time and replies!

Keyan Abtahi
Python Web Development Techdegree Student 267 PointsEric - thank you so much. I can't thank you enough for your time and effort! I finally learned how to do this - it's been holding me.back.immensely! Thank you!

Keyan Abtahi
Python Web Development Techdegree Student 267 PointsHello Eric, thank you for your reply! That makes sense, your example is great!
A last question regarding this is, what if its reversed? What if you want to take something defined in a functions scope and use it outside the said function?
E.g: https://pastebin.com/J17xF2FU
Thank you!