Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial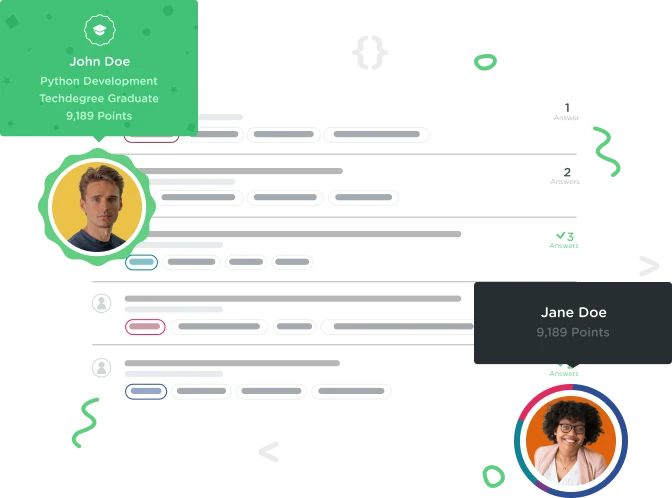
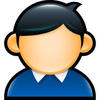
Daniel Hildreth
16,170 PointsBecome Zen C# Streams Challenge 2
I am stumped at the last part of this question. I am not sure what I'm doing wrong. Can someone help and explain this to me?
The question asks:
In the BecomeZen method, create a new WebClient object named webClient. Remember that WebClient is disposable, so wrap it in a using statement. We'll need to add a user-agent header, which specifies the "agent" that is sending the request. In most cases, it's a browser, but in our case, it's this code challenge! Inside the using block, add a Header with a name of "User-Agent" and a value of "CodeChallenge".
using System;
using System.IO;
using System.Net;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine(BecomeZen());
}
public static string BecomeZen()
{
string zenResponse = "";
using(var webClient = new WebClient())
{
webClient.Headers.Add("User-Agent", "CodeChallenge");
string response = webClient.DownloadString(string.Format("https://api.github.com/zen", zenResponse));
}
return zenResponse;
}
}
}
5 Answers
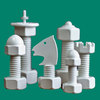
Steven Parker
231,269 Points
Your first try has an invalid call to string.Format:
string.Format("https://api.github.com/zen", zenResponse)
While this may not generate a compiler error, you can see that there is a second argument to be included in the format but the format string has no placeholder ("{0}
") to put it into. At this point, zenResponse is just an empty string so it would not make sense to include it anyway.
As you realized, only the plain string itself is needed as the argument to DownloadString.
However — there's an even more serious error here!
The challenge failed to notice that you did not pass back the collected information. Look at this line:
string response = webClient.DownloadString("https://api.github.com/zen");
You are creating a new variable "response" here which is then immediately disposed as you exit the using. The function would always return an empty string! But what the challenge specifies would look like this instead:
zenResponse = webClient.DownloadString("https://api.github.com/zen");
Then, zenRespose is returned by the function.
You might want to report this as a bug on the Support page. It may get you an Exterminator badge!

Sara Rena Anderson
15,045 Points`` using System; using System.IO; using System.Net;
namespace Treehouse.CodeChallenges { class Program { static void Main(string[] args) { Console.WriteLine(BecomeZen()); } public static string BecomeZen() { string zenResponse = "";
using(var webClient = new WebClient())
{
webClient.Headers.Add("User-Agent", "CodeChallenge");
zenResponse = webClient.DownloadString("https://api.github.com/zen");
}
return zenResponse;
}
}
} ``

Sanele Mogale
1,075 PointsSteven's method is not working too. I think there might be something wrong coz I struggled and ended up using Steven's method with no luck
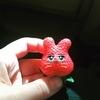
Mariia Ashurova
10,913 Pointsusing System; using System.IO; using System.Net;
namespace Treehouse.CodeChallenges { class Program { static void Main(string[] args) { Console.WriteLine(BecomeZen()); } public static string BecomeZen() { string zenResponse = "";
using(var webClient = new WebClient())
{
webClient.Headers.Add("User-Agent", "CodeChallenge");
string response = webClient.DownloadString("https://api.github.com/zen");
}
return zenResponse;
}
}
}

James Churchill
Treehouse TeacherDaniel,
Thanks so much for reporting this issue to our support. And thanks to Steven for his excellent review and feedback!
I'm happy to report that this issue has been resolved in this code challenge.
Thanks ~James
Daniel Hildreth
16,170 PointsDaniel Hildreth
16,170 PointsI passed this challenge by passing this code into it. It is very similar to what I previously had. Can someone please explain the differences between my two codes? The failed way kept wanting me to pass in that GitHub address to the DownloadString method.