Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial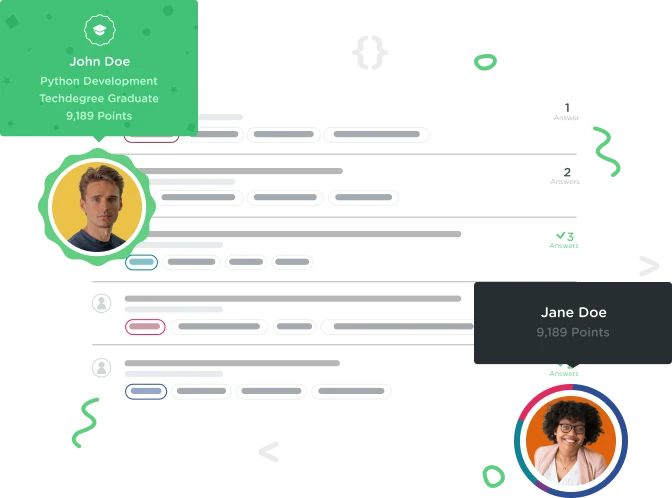

yoav green
8,611 Pointsbeen trying to log another "getter" with no succes
class Pet {
constructor(animal, age, bread, sound){
this.animal = animal;
this.age = age;
this.bread = bread;
this.sound = sound;
}
get activity() { //getter method//
const today = new Date();
const hour = today.getHours();
if (hour > 8 && hour <=20) {
return 'playing';
} else {
return 'sleeping';
}
if (hour > 16 && hour <=19) {
return 'meeting the Bros in the hood';
} else {
return 'at home, board to death';
}
}
speak() {
console.log(this.sound);
};
}
const ernie = new Pet('dog', 1, 'pug', 'woof woof');
const vera = new Pet('cat', 5, 'persian', 'meouuu');
console.log(ernie.activity);
4 Answers

Siddharth Pande
9,046 Points// I think this is what you were trying to do......
class Pet {
constructor(animal, age, bread, sound){
this.animal = animal;
this.age = age;
this.bread = bread;
this.sound = sound;
}
get activity() { //getter method//
const today = new Date();
const hour = today.getHours();
if (hour > 8 && hour <=15) {
return 'playing';
} else if (hour >= 16 && hour <=19) {
return 'meeting the Bros in the hood';
} else {
return 'at home, board to death';
}
}
speak() {
console.log(this.sound);
}
}
const ernie = new Pet('dog', 1, 'pug', 'woof woof');
const vera = new Pet('cat', 5, 'persian', 'meouuu');
console.log(ernie.activity);
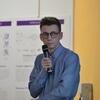
Dima Fedin
7,651 PointsYour second conditional statement will never work:
// your code...
get activity() {
const today = new Date();
const hour = today.getHours();
if (hour > 8 && hour <=20) { // JS checks this condition. If it's true it will return 'playing' else...
return 'playing';
} else { // ... else JS just goes to this block and returns 'sleeping'
return 'sleeping';
}
/*
That's all. Your code will never continue to second conditional block
because when function returns anything it stops execution.
*/
if (hour > 16 && hour <=19) {
return 'meeting the Bros in the hood';
} else {
return 'at home, board to death';
}
}
// your code...
You can solve this problem like this:
// your code...
get activity() {
const today = new Date();
const hour = today.getHours();
const activities = [];
if (hour > 8 && hour <=20) {
activities.push('playing');
} else {
activities.push('sleeping');
}
if (hour > 16 && hour <=19) {
activities.push('meeting the Bros in the hood');
} else {
activities.push('at home, board to death');
}
return activities;
}
// your code...

Jackson Monk
4,527 PointsWhen I run this, it logs 'playing' to the console. Also, what time did you run this? Your second if statement would only run if it was between 4:00p.m. and 7:00p.m.

yoav green
8,611 Pointsit ran in the time of the condition. i want it to log playing and the second conditional statement