Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial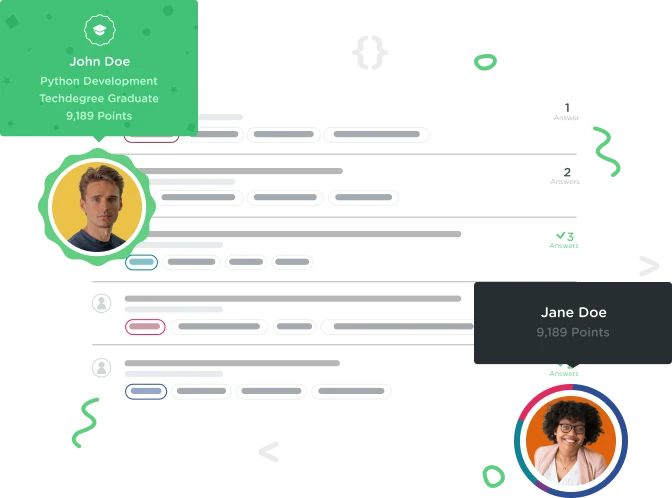
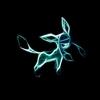
Randall Varasteh
Python Development Techdegree Student 2,814 PointsBeginner Question
Hi I'm learning Python as my first language and am new to computer science in general. Most of what is being talked about is easy to understand, but I'm not exactly sure how the "." works. I've seen it starting to be used a lot in different ways like class.method() but was looking for a more clear, general definition of what the . is and what it is doing. If anyone can provide an answer or a link to some documentation on it that would be very helpful. Thank you.
1 Answer
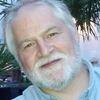
Jeff Muday
Treehouse Moderator 28,720 PointsThis is a great question to ask.
In short, the dot is a syntactic element that separates a "namespace." A namespace is a hierarchy in Python. In Python, everything is an object-- don't worry if this doesn't make sense now, but you will learn more about this as you go further in your studies. So a namespace is closely related to Python's object-orientation.
In the first example, we will import a library (or package) namespace.
Here, I can import math, and then get the value of "pi" from that namespace.
import math # import a library (or namespace)
print(math.pi) # prints out 3.14159
The namespace might be associated with an instantiated object. In this case, the dot gives access to a named property or access to a method (or function of the object).
class Person:
# this is an object with one property and one method
name = 'George Washington'
def greet(self):
print("Hello " + self.name)
x = Person() # instantiate the Person object as x
print(x.name) # prints out George Washington
x.greet() # prints "Hello George Washington"
Good luck with your Computer Science studies. Python isn't the only language you will learn along the way, but could be the most important depending on your career path.
Randall Varasteh
Python Development Techdegree Student 2,814 PointsRandall Varasteh
Python Development Techdegree Student 2,814 PointsIt being a hierarchy makes a lot more sense, all this was copied to my notes. thank you!