Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial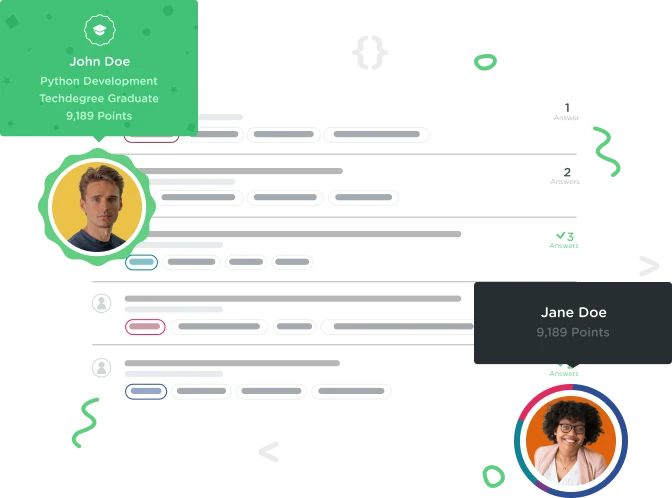

Artur Owczarek
4,781 PointsBeginning Python - sillycase
I got this error: Traceback (most recent call last): " File "C:/Users/Artur/Desktop/Python/Sillycase/sillycase.py", line 12, in <module> print(sillycase("Treehouse")) File "C:/Users/Artur/Desktop/Python/Sillycase/sillycase.py", line 4, in sillycase word_copy[index] = word_copy.lower() AttributeError: 'list' object has no attribute 'lower' "
But I don't understand. In my opinion word_copy[index] isn't a list but a string.
def sillycase(word):
word_copy = list(word)
for index in range(len(word) // 2):
word_copy[index] = word_copy.lower()
for index in range(len(word) // 2 + 1, len(word) - 1):
word_copy[index] = word_copy.upper()
word = ""
for letter in word_copy:
word += letter
return word
3 Answers
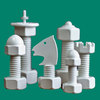
Steven Parker
231,275 PointsBut "word_copy" is indeed a list. It is created by converting "word" into a list on the very first line:
word_copy = list(word)
But you don't need to convert it, since slices work on strings just as well as they do on lists. And the whole purpose of this exercise is to practice using slices. Try rethinking your approach to make use of them, which will also save you from needing any loops.

Artur Owczarek
4,781 PointsThank you very much for a help!
I wrote the code in that way and it works. :D
def sillycase(word):
lower = word.lower()
upper = word.upper()
return lower[:len(word) // 2] + upper[len(word) // 2:]
Anyway, I don't understand why in the previous code word_copy[index] is a list. It's weird. Components of word_copy are one character strings so why word_copy[index] is a list? :)
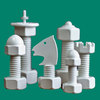
Steven Parker
231,275 PointsYou're right, word_copy[index]
is not a list, since it's just one item. But where the code has: "word_copy.lower()
", the "lower" method is being applied directly to "word_copy" itself, which is a list. And that causes the error.
Happy coding!

Artur Owczarek
4,781 PointsAhhh I got it. Thanks very much again. :)
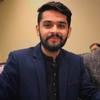
Junaid Abbasi
3,518 Pointsthis is so easy to understand!!

Neil Brown
22,514 Pointsword_copy[index] is a string. word_copy is a list (of string characters). The error comes from you applying lower() to word_copy, which is a list.
Alvand Hajizadeh
2,069 PointsAlvand Hajizadeh
2,069 PointsReally cool to see how you built this! How does python know what you mean by "letter" since it is not defined anywhere in the function?