Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial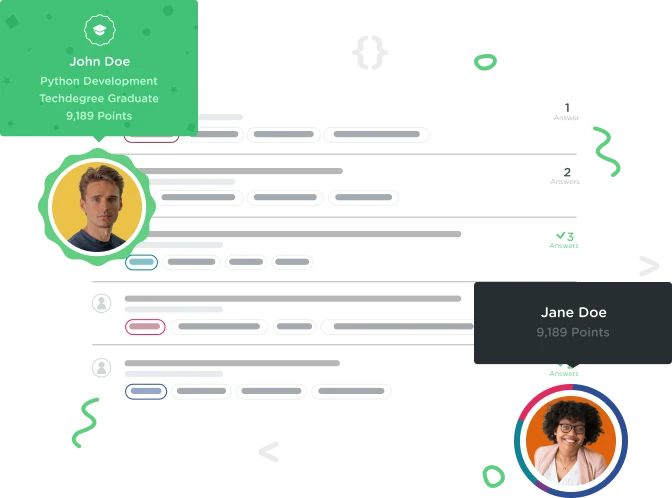
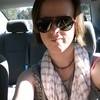
karis hutcheson
7,036 PointsBenefit(s) of using private methods in this case vs. passing in an argument directly?
I was just noticing that Craig was using private methods to pass in our "#" and "@" characters to the getPrefix() function like:
private List<String> getWordWithPrefix(String prefix) {
List<String> results = new ArrayList<String>();
for ( String word : getWords() ) {
if (word.startsWith(prefix) ) {
results.add(word);
}
}
return results;
}
public List<String> getHashtags() {
return getWordWithPrefix("#");
}
//Then calling Treet.getHashtags();
and I was thinking it might be more DRY to just make call the function from our Example class and pass in the character argument like so:
public List<String> getSpecials(String character) {
List<String> results = new ArrayList<String>();
for ( String word : getWords() ) {
if (word.startsWith(character) ) {
results.add(word);
}
}
return results;
}
//Then calling Treet.getSpecials("#");
I feel like if, at some point, Twitter were to change the way you tag people or add another special prefix then I would need to edit both my Treet class and my Example class to cope with the change whereas the latter method just requires changing the function argument in one place. Am I way off base? What would be the benefits of using the private method?
1 Answer

Craig Dennis
Treehouse TeacherHi Karis!
Great question! We're definitely on the same wave length.
Let me explain my thinking a bit.
I used private, because I didn't want to expose that method to callers, as it wouldn't make too much sense. getHashtags
and getMentions
definitely benefit from the method, but if those ever change like you mentioned, I can change the exposed method.
Let's walk it a bit if they start using $
for mentions, instead of @
for instance, if we had exposed the ability to getSpecial
characters, that could be used all over the place and is out of our control. Any code would/could arbitrarily call that, and we wouldn't know.
On the flip side with it hidden, we can change the getMentions
to use the new $
but also support the @
and it would still be backwards compatible. We also have the added benefit of improving the way we get these, maybe we no longer want to use a Regex but use a better way. All of this is up to us, because we only exposed what they want to change.
Excellent way of thinking about it, I see your point too, keep those coming!