Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial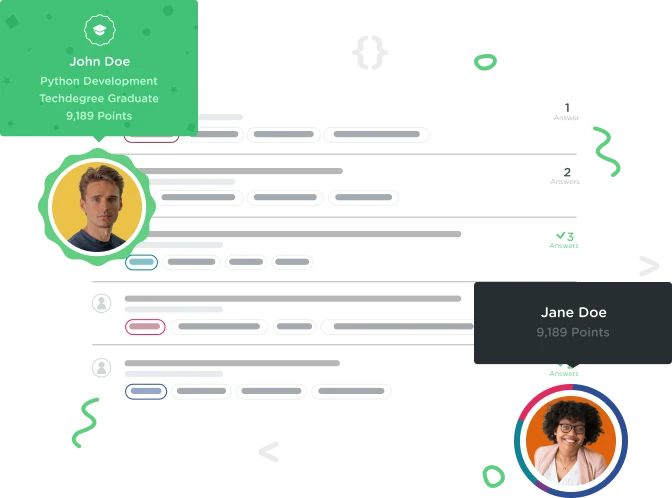

Quicken Loans
12,654 PointsBest approach to insert classes based on current page
So I'm using an unordered list for site navigation like so:
<nav>
<ul>
<li><a href='page1.html' class='currentPage'>Page 1</a></li>
<li><a href='page2.html'>Page 2</a></li>
<li><a href='page3.html'>Page 3</a></li>
<li><a href='page4.html'>Page 4</a></li>
</ul>
</nav>
I am using a class to bold the page that the user is currently on like so:
.currentPage {font-weight:bold;}
My question is how can I insert this class automatically when the user navigates between pages? Can this be done using PHP or would it be more of a JS thing?
I am thinking it would look something like this
<li>< a href='page1.html'
<php?
If the current page is page1.html {
echo "class=current"
};
?>
>Page1</a></li>
I hope I explained this clearly. Any advice would be appreciated :)
3 Answers

Liz Karaffa
17,576 PointsYou could use either PHP or JS/jQuery to add the class.
If you're using PHP on every page at the top of the page in PHP tags you can define a variable to the page name. i.e on the home page have $page= "page1";
on page 2: $page= "page2";
on page 3: $page= "page3";
etc.
Then in your unordered list do something like this
<nav>
<ul>
<li class='<?php if ($page == "page1") { echo "currentPage"; } ?>'><a href='page1.html' >Page 1</a></li>
<li class='<?php if ($page == "page2") { echo "currentPage"; } ?>'><a href='page2.html'>Page 2</a></li>
<li class='<?php if ($page == "page3") { echo "currentPage"; } ?>'><a href='page3.html'>Page 3</a></li>
<li class='<?php if ($page == "page4") { echo "currentPage"; } ?>'><a href='page4.html'>Page 4</a></li>
</ul>
</nav>
EDIT: Sorry just realized that below would add a class when you click on something, not when you're on the current page.
If want to use jQuery, don't have any classes in your <li>
and then add them with jQuery.
var selector = "nav li";
$(selector).click(function(){
$(selector).removeClass('currentPage');
$(this).addClass('currentPage');
});
See my codepen of the jQuery example in action http://codepen.io/anon/pen/hyaAw
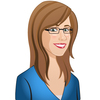
Shaker Advertising
5,395 PointsTo do this dynamically you can use a little jQuery to add class current to the nav item based on if it's href matches the current URL string:
<nav>
<ul>
<li><a href="/about/">About Us</a></li>
<li><a href="/work/">Our Work</a></li>
</ul>
</nav>
$(function() {
$('nav a[href^="/' + location.pathname.split("/")[1] + '"]').addClass('current');
});
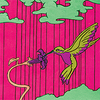
Christopher Bijalba
12,446 Pointsalso you can use php with the methodology denoted by connor, you do not need to add page variables as offered by liz, though that works just fine.
you can use php to simply get the page you're on and insert that into liz's if statements, via checking $_SERVER array: