Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial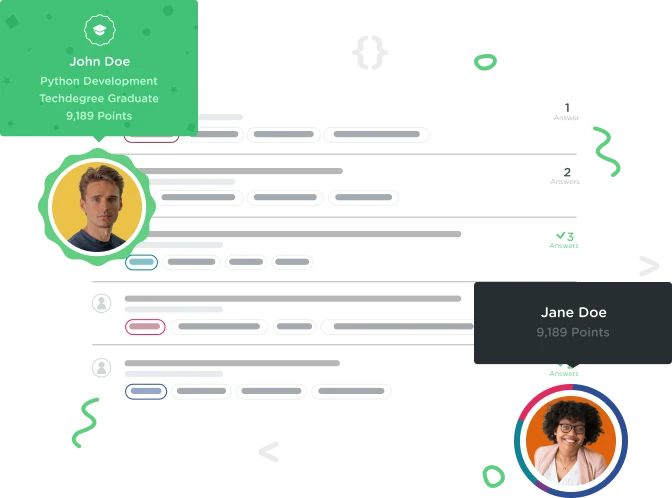
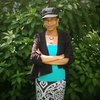
Ivy W.
7,427 PointsBest practices for variable scope with loops?
So I think I managed to get the challenge on the first try (this is pretty novel for me since starting the JavaScript courses) and below is my code:
var html = '';
var red;
var green;
var blue;
var rgbColor;
for (i = 0; i <= 10; i++ ) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
document.write(html);
My question refers back to the videos on scope for variables. I declared mine outside of the for loop when I initially did this and it worked, but I'm wondering if that's bad practice for loops. Can anyone tell me if there's any specific guidelines when declaring variables when using loops?
After looking at the video involving functions, loops, and scope near the end of the course, I think I understand why it's important to do so in a function, but I'm not sure if loops follow the same protocol.
2 Answers
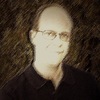
Jason Anders
Treehouse Moderator 145,860 PointsHi Ivy,
I don't feel loops follow that same convention. The way you declared the variables is the way I would and the way I've seen it done. You really don't want to clutter up your loop with declarations, and without declaring the variables first, the loop wouldn't work (you'd end up with an 'undefined' error). You could declare them inside of the loop, but for readability and what is the 'norm', I would declare them outside of the loop.
I did notice that you didn't declare the 'i' variable used in the loop. And you said that it still worked? I know it's been a bit since I've done JavaScript, but I thought the loop needed to be for (var i = 0; i <= 10; i++)
. Without declaring it in the loop with var
, it should have thrown an error.
Good job though! Keep Coding. :)
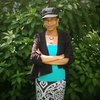
Ivy W.
7,427 PointsHi Jason,
That makes a lot of sense, thanks! I think I'm going to go back and review that lesson (I'm also using Jon Duckett's JavaScript & JQuery book so I may be getting the topics mixed up). Also, I just noticed what you said about my for loop. I think I accidentally cut it out while I was C & P, but I did declare the variable in the workspace. Thanks for pointing it out!