Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial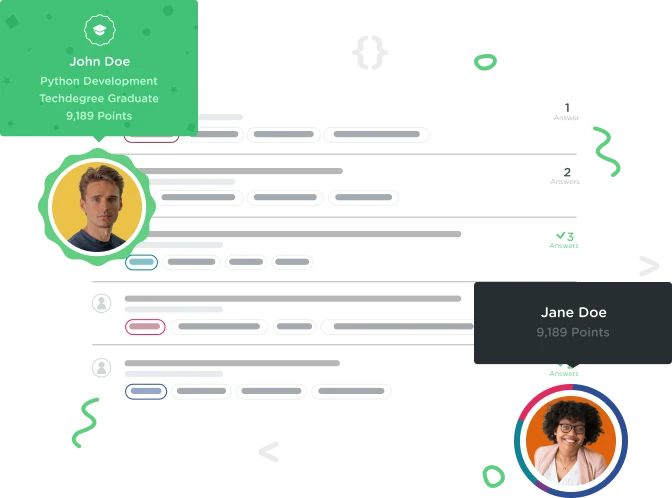
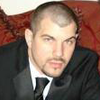
Gary Calhoun
10,317 PointsBest way to generate checkboxes and questions for multiple choice quiz
Need some direction with this. I have objects in an array and I want to generate the checkboxes and questions and what user chooses I am using mobile-jquery plugin for the checkboxes
//User clicks button to have the quiz application pop up using jquery
$('button').click(function(){
$('#quiz').slideDown();
$(this).hide();
$(".btn-primary").css("cssText", "display: block !important; margin:auto; width: 30%;");
});
var score = null;
var questionNumber = 0;
var questions = [
//Question 1
{
'answer': 'b',
'question': 'What is Apple\'s most lucrative product of 2015?',
'options': ['Iphone', 'Apple Watch', 'Ipad']
},
//Question 2
{
'answer': 'a',
'question': 'Who invented the tablet?',
'options': ['Microsoft', 'Google', 'Apple']
},
//Question 3
{
'answer': 'b',
'question': 'In 1999 who created the first mp3 phone?',
'options': ['Toshiba', 'Samsung', 'Sony']
},
//Question 4
{
'answer': 'a',
'question': 'Which tech company released The Walkman?',
'options': ['Sony', 'Samsung', 'Toshiba']
},
//Question 5
{
'answer': 'b',
'question': 'Which company created the "slide to unlock" on smartphones?',
'options': ['Apple', 'Microsoft', 'Google']
},
//Question 6
{
'answer': 'c',
'question': 'Who invented the holographic computer known as the HoloLens?',
'options': ['Google', 'Apple', 'Microsoft']
},
//Question 7
{
'answer': 'c',
'question': 'NAND Flash Memory was created by which tech company?',
'options': ['Sony', 'Samsung', 'Toshiba']
},
//Question 8
{
'answer': 'b',
'question': 'Who first invented RDF Site Summary (RSS)?',
'options': ['Lycos', 'Netscape', 'Yahoo']
},
//Question 9
{
'answer': 'b',
'question': 'Which company released their TV Product first?',
'options': ['Roku', 'Apple TV', 'Chromecast']
},
//Question 10
{
'answer': 'b',
'question': 'Who created the first Motion Controller?',
'options': ['Nintendo', 'Microsoft', 'Sony']
}
];
//maybe the questions fly in or something similar?
//Questions are then presented
//If wrong display sorry that is the wrong answer but here is the right one
//Maybe an x or a graphic can pop up when they get it wrong
//maybe use modal for pop ups of right or wrong
//if right display you got the correct answer! maybe a green check mark or a thumbs up
//Thank user for taking quiz
//click to add the scores
//If all 10 correct show jquery confetti animation "Wow you got all the answers right!"
//if all wrong display some other animation?? "Sorry you got all the answers wrong"
//prompt play again maybe?
//if some correct have a different animation "You did ok"
//pop up a social reshare for facebook twitter and google maybe so others can share the app
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="description" content="">
<meta name="author" content="">
<title>Corporate Tech Giants Quiz</title>
<!-- Quiz CSS -->
<link href="css/quiz.css" rel="stylesheet">
<!-- Bootstrap Core CSS -->
<link href="css/bootstrap.min.css" rel="stylesheet">
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/libs/html5shiv/3.7.0/html5shiv.js"></script>
<script src="https://oss.maxcdn.com/libs/respond.js/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<!-- Page Content -->
<div class="container">
<div class="row">
<div class="col-lg-12 text-center">
<h1>Corporate Tech Giants Quiz</h1>
<button type="button" class="btn btn-lg btn-default"><span class="glyphicon glyphicon-arrow-right"></span>Get Started</button>
<div id="quiz">
<div id="question">
<div id="questions">
<label><input type="radio" value="a"></label>
<label><input type="radio" value="b"></label>
<label><input type="radio" value="c"></label>
</div>
</div>
<button type="button" class="btn btn-primary">Submit</button>
</div>
</div>
</div>
<!-- /.row -->
</div>
<!-- /.container -->
<!-- jQuery Version 1.11.1 -->
<script src="js/jquery.js"></script>
<!-- Bootstrap Core JavaScript -->
<script src="js/bootstrap.min.js"></script>
<script src="js/quiz.js"></script>
<script src="http://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.js"></script>
</body>
</html>
2 Answers

James Kim
8,475 PointsHey Gary... I'm not sure if this is what you are asking for, but I would also make an empty array for answers that users pick so it can get stored in there and also i would do if else statements... so like..
if(answers === radioButton.values()){
console.log("answer is correct!");
}else{
console.log("incorrect answer");
}
or something like that...
Also it seems like you want to have some animation... so suggest that you should check out animate.css and use JQuery's addClass and removeClass methods.
Hope that helped!.

Alex Pritchett
3,470 PointsHi Gary, I just stumbled across your question and have been working on a similar project myself. Have you been able to make much progress with this? If so, I'd love to hear about the solution that you went with.
Gary Calhoun
10,317 PointsGary Calhoun
10,317 PointsThanks, I am probably going to scratch mobile jquery checkbox generation because its clashing with the bootstrap I am using for this practice application and was giving me doubles of my buttons. I will look into animate.css , thanks for the code I will mess around and see if I can make it work. I looked at several online examples here is a list at some I came across
This is the one I was trying to follow originally but the code wasn't working http://docs.codiqa.com/tutorials/multiple-choice-quiz/
http://code.tutsplus.com/tutorials/build-a-spiffy-quiz-engine--net-20185
http://www.raymondcamden.com/2013/12/5/Building-a-Quiz-Manager-for-jQuery-Mobile
http://www.scribd.com/doc/198938320/HTML5-Multi-choice-Quiz-Tutorial#scribd
http://jsfiddle.net/nhxuy/
http://jsfiddle.net/tilwinjoy/tzXgM/1/
I guess all in all I am trying to build something a bit more advanced then what I have learned on treehouse, I will look at some more examples and do so more research.