Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial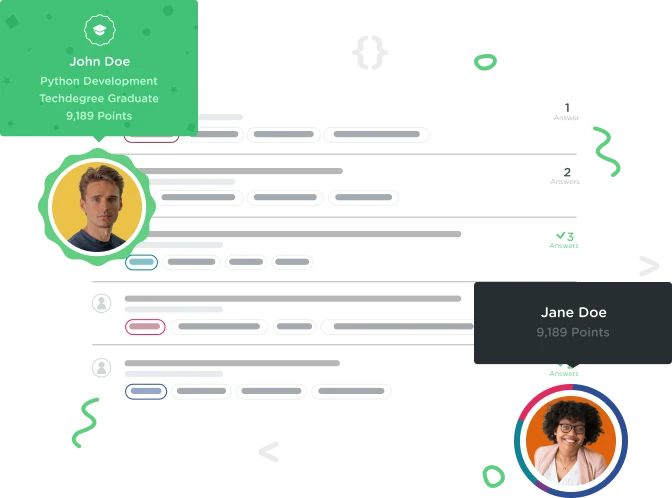
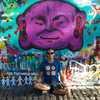
Andrew Stelmach
12,583 PointsBest way to remove punctuation from a String?
Extra Credit challenge: remove all punctuation from a string and print it in reverse.
puts "Give me a string. "
string = gets.gsub(/[!@#$%^&*()-=_+|;':",.<>?']/, '')
puts string.reverse
This works, but it's not very elegant. Must be a generic term for 'punctuation' I can use inside the gsub?
7 Answers

Amber Taniuchi
4,579 PointsSo I was thumbing through the link that Telmen posted and found \W (uppercase W) which does the same thing that [^A-Za-z0-9_] does.
string.gsub(/\W/, ' ')
worked just as beautifully for me :)
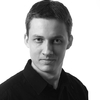
Maciej Czuchnowski
36,441 PointsYou could use a shorter regex that simply accepts only uppercase and lowercase letters, numbers and spaces:
string.gsub(/[^A-Za-z0-9\s]/i, '')
his way you don't have to fear that you forgot about some particular character.
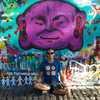
Andrew Stelmach
12,583 PointsThanks! That works a treat! My code:
puts "Give me a string, fool. "
string = gets.gsub(/[^A-Za-z0-9\s]/i, ' ')
puts string.reverse
Could you please tell me WHY this works though? Explain what the different characters mean?
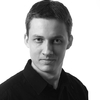
Maciej Czuchnowski
36,441 PointsA-Z - uppercase letters, a-z - lowercase letters, 0-9 - numbers, \s - spaces. You can play with Ruby regexes here to make sure your code does what you want aside from testing it in your own program:
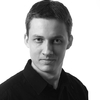
Maciej Czuchnowski
36,441 PointsThe ^ at the beginning makes it the opposite of what you did in the question. The i at the end probably is not necessary, but make sure you test it properly.

Chris Shaw
26,676 PointsHi Andrew,
The code you have above is really the only way to remove specific parts of a string and what you have is as elegant as regular expressions get.
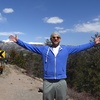
gerald blady
9,052 PointsI used this for the challenge. The .chop command. It seemed to work as it removed the "!" from the end.
string = "this is a string!"
puts string.chop
puts string.chop,reverse

Telmen Davaadorj
4,251 PointsIn general, Try to play with http://www.regexr.com/ and find the pattern you want to put in gsub method.
Tnx

Unsubscribed User
3,309 PointsI didn't like that my (liberal) punctuation was being replaced by the odd extra space (say, after a period). I used a POSIX regexp and added squeeze:
str.gsub(/[[:punct:]]/,'').squeeze(' ')
erik oseland
357 Pointserik oseland
357 PointsAmber! You saved me so much time! Thanks. Best answer so far. P.S. I was just through Salt Lake City. So beautiful...