Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial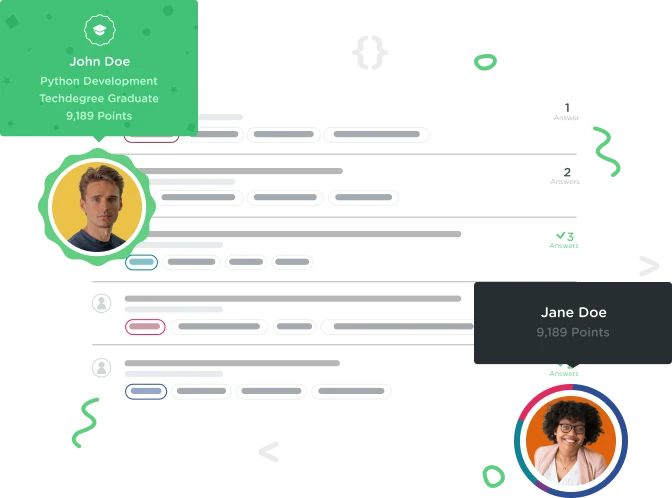

Howie F
1,710 PointsBetter explanation/tutorial for initializers?
Can someone please point me (a beginner with basically no programming experience) to a better explanation/tutorial to help me understand initializers? I've listened to Amit's videos 2-3 times and still have no idea why they are needed, when different types of initializers should be used, etc. I think Amit is omitting some basic notions.
For example, he doesn't offer a precise definition of the "self" keyword. I'd like to see a precise definition along with its use in a statement as such:
self.sides = sides [1] [2] [3]
[1] = definition of "self" keyword and what its function is [2] = definition of "sides", and what it's referring to [3] = definition of "sides", and what it's referring to
Thank you!
3 Answers
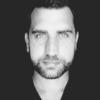
Jhoan Arango
14,575 PointsHello Howie :
The following paragraph is from the e-book The Swift Programming Language and I recommend downloading it in iBooks.
Initialization is the process of preparing an instance of a class, structure, or enumeration for use. This process involves setting an initial value for each stored property on that instance and performing any other setup or initialization that is required before the new instance is ready for use
Apple Inc. “The Swift Programming Language (Swift 2 Prerelease).” iBooks. https://itun.es/us/k5SW7.l
What does that mean ? Let’s see it in code.
/*
We are going to create a class, and this class has
three store properties.
*/
class CarFactory {
let wheels: Int
let doors: Int
let colors: String
}
/*
Currently this class has no initializers or initial values, therefore we can not
create an instance of it. We have to give those store properties
some values, by means of an initilizer.
*/
class CarFactory {
let wheels: Int
let doors: Int
let colors: String
init(wheels: Int, doors: Int, colors: String) {
self.wheels = wheels
self.doors = doors
self.colors = colors
}
}
// There we have an initializer, but what does that do ?
/*
When creating an instance of the class CarFactory, we will
give values to the store properties, therefore we will successfully
create an instance.
*/
Notice how self is used to distinguish the name property from the name argument to the initializer. The arguments to the initializer are passed like a function call when you create an instance of the class.
Apple Inc. “The Swift Programming Language (Swift 2 Prerelease).” iBooks. https://itun.es/us/k5SW7.l
/*
The word self is use here so that you can distinguish a property,
from a named argument. I’m going to change the name of one of the parameters
in the initializer, so that you can see the difference.
*/
class CarFactory {
let wheels: Int
let doors: Int
let colors: String
init(wheels: Int, doors: Int, chooseColor: String) { // see the change?
self.wheels = wheels
self.doors = doors
colors = chooseColor // the word self is not required here
}
}
/*
The word self is not required there since the name of the parameter
is different from the name of the property. So the system knows which
is which.
*/
Now let’s create that instance
// Instance
let fordFiesta = CarFactory(wheels: 4, doors: 2, colors: "Black”)
// Now you can access the properties of this instance like this
fordFiesta.wheels // this will show 4
fordFiesta.doors // this will show 2
// we can also use the print method
println(fordFiesta.color) // this will print “Black"
Take it one step at a time, re-watch the videos as many times, read the book from apple, and trust me, little by little you will start making sense of everything, I hope this gives you a different view of initializers and you are able to continue your learning.
Good luck.

Howie F
1,710 PointsJhoan,
Thanks for responding. I'm going through the "iOS Development with Swift" track sequentially and am currently in the "Object Oriented Swift" section.
Howie
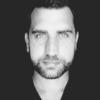
Jhoan Arango
14,575 PointsHello :
To answer your questions I will go one by one..
Answer to Question 1
Yes, that is correct.
class CarFactory {
let wheels: Int <---- Refers to this
let doors: Int
let colors: String
init(wheels: Int, doors: Int, colors: String) {
This ---> self.wheels = wheels
self.doors = doors
self.colors = colors
}
}
class CarFactory {
let wheels: Int
let doors: Int
let colors: String
// here
init(wheels: <-- Refers to this Int, doors: Int, colors: String) {
self.wheels = wheels <--- This
self.doors = doors
self.colors = colors
}
}
Answer to Question 2
Initializing is GIVING a constant or a variable a value.
var someVariable: String // has no initial value
var someVariable: String = "Value" // This one has an initial value
/*
Here we are declaring a new variable called “ someVariable” of
type String. BUT it does not have ANY value. We can initialize it by
giving it a value by means of an initializer.
*/
func AnyFunction(someVariable: String) {
var someVariable: String <-- has no value //
init(someVariable: String) {
self.someVariable = someVariable
}
}
let instance = AnyFunction(someVariable: "Value") <-- giving it a value //
instance.someVariable // This is how we access the value
Answer to Question 3
Yes you got it. That is exactly how the instance would look like!
Answer to Question 4
1) Initializer is what is used to give values to store properties in a class.
2) Initial value is the first value you assign to a constant or variable
3)
class CarFactory {
let wheels: Int = 4 // Default
let doors: Int
let color: String
init(doors: Int, color: String) {
self.doors = doors
self.color = color
}
}
let fordFiesta = CarFactory(doors: 2, color: "Black”) // “wheels” is not here
fordFiesta.wheels // but we can still access “wheels” here and it will return 4
fordFiesta.color
fordFiesta.doors
Notice how let wheels: Int = 4 has a value already ? Well since it already has a value it becomes a default value, and it no longer needs to be initialized by the initializer. But when you create an instance of CarFactory, you can then access the value of “wheels”.
Now if all of the store properties had “Default values” Then we would not need an initializer method.
class CarFactory {
let wheels: Int = 4
let doors: Int = 2
let color: String = "Black"
// No initializer method
}
let fordFiesta = CarFactory() // No need of initializer
// But we can still access those “default” values.
println(fordFiesta.wheels) // this will print 4
println(fordFiesta.color) // this will print “Black”
println(fordFiesta.doors) // this will print 2
Answer to Question 5
This question is answered by all of the above answers.
I hope you understand now.
Good luck :)

Howie F
1,710 PointsJhoan,
This is much clearer to me now. Thanks for taking the time to explain this to me! Very helpful.
Howie F
1,710 PointsHowie F
1,710 PointsThank you very much Jhoan. This is very helpful!
I probably still have this wrong, so a few questions:
Sorry for all the questions, I do appreciate it!
Jhoan Arango
14,575 PointsJhoan Arango
14,575 PointsHello, I will answer your questions once I'm in a laptop, I'm currently on my phone and is a pain to type on here.
But I'd like to know something before I know how to answer your questions. Have you gone through the whole swift course ? From the beginners part ?
I ask you this because I want to know how detailed I have to be with my answer.
Thanks