Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial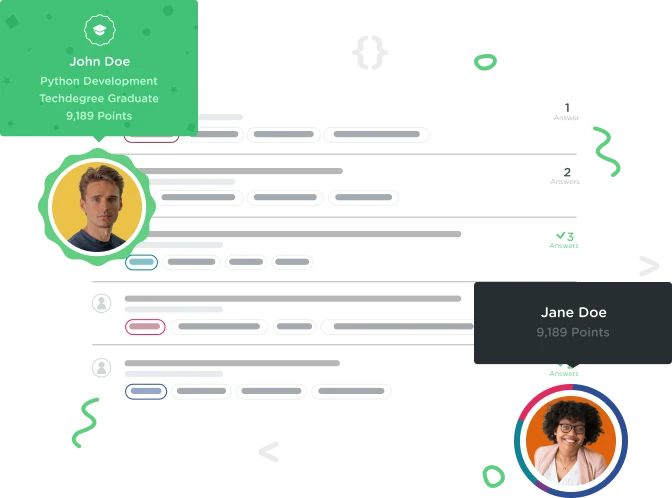

joshuawalliy
1,131 PointsBetter Help?
I need some help.
- I don't understand what return is needed for. I understand how to make functions I just don't understand why we need returns and how to make them could someone help me. Also, I am wondering what might be wrong with my code. Thanks, Josh
func greeting(person: String,hello: String )-> string {
return hello, person
}
greeting(Tom,Hello)
1 Answer
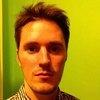
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsNot all functions return something. Sometimes they just perform an action inside the function, which is the case with the function they gave you to start with that had "println("Hello (person))".
There are a few things wrong with your code:
1) It should take only one argument: (person: String)
. That's the part that is going to change depending on the name of the person you pass in. The word "hello" is always going to be the same.
2) The "string" that you specify as the return value needs to be capitalized: " -> String "
3) when you return the string inside the function, it needs to be in quotes, and you need to use string interpolation for the "person" variable - like this
return "Hello \(person)"
Also, the word "Hello" needs to be capitalized. The swift language doesn't care, but this code challenge does. They also want the phrase without a comma.
The whole thing should look like this:
func greeting(person: String) -> String {
return "Hello \(person)"
}
The advantage of having a function return something is that you can you that returned value and put it into a variable, or you can pass it into another function. That way your function could be part of a chain of events.
So you could do something like this later:
var greetingOutput = greeting("Dave") // This takes "Hello, Dave" and puts it inside the variable 'greetingOutput'
println(greetingOutput) // this takes that string you made and passes it into the println function
chunchang pan
4,319 Pointschunchang pan
4,319 PointsYou can rewrite your code like below. I think this is what you want. func greeting(person: String,hello: String )-> String { return "(hello), (person)" }