Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial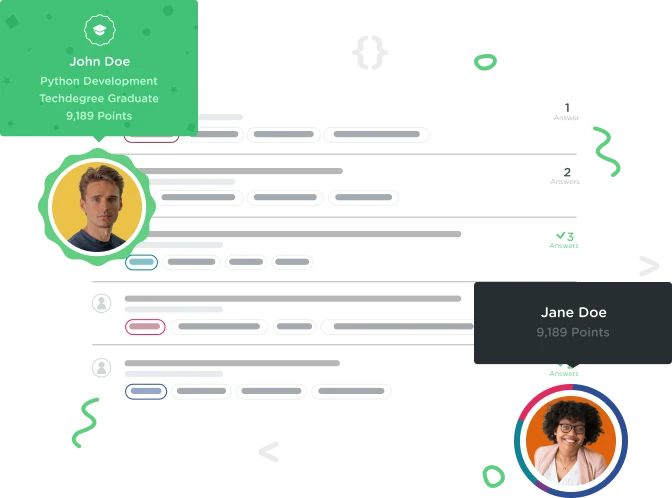

jessicawynn2
7,971 PointsBetter way of receiving input from users?
I misunderstood the assignment a bit and thought I was supposed to get both numbers from the user through a prompt. Since he did not go over it in the video, what would be a good way to take 2 sets of information to run through a function at the same time? My code seems to "work" but I would like to know a better/proper way to do it without using the console.log.
Also, the result that I get does not seem so random. Am I truly getting a random number from my code?
//Creates a random number between 2 numbers provided by the user
function randomNumber() {
if(number1 > number2) {
return Math.floor(Math.random() * (number1 - number2 + 1)) + number2;
} else if (number1 < number2) {
return Math.floor(Math.random() * (number2 - number1 + 1)) + number1;
} else if (number1 === number2) {
alert("You typed the same number.")
} else {
alert(prompt("Uh oh! That wasn't a number!"));
}
}
alert("This program provides a random number between the two numbers you provide");
var number1= parseInt(prompt("Type the first number."));
var number2= parseInt(prompt("Type the second number."));
document.write(randomNumber());
4 Answers
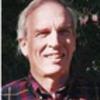
jcorum
71,830 PointsNot sure I understand your question. But re a "good way" to get info from a user, alerts and prompts don't really do much for me. Too slow and painful. It would be a much nicer user experience if you had two input text boxes the user could enter the numbers in at one time, and some element on the page (like a p or a span) you could write the answer to. But that would be way beyond the scope of the challenge.
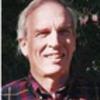
jcorum
71,830 PointsAfter I sent the above, I thought that rather than saying what should be done, I should try to do it. So here's a stab at what I was suggesting. I put the javascript in the html, which I know is frowned on. But I couldn't get the Workspace to update the js. Overtime I looked it was still the original Treehouse formula. So if you know how to do that I'd appreciate a tip! Also, I didn't do anything with the CSS, so it looks a bit weird. But the idea was to get user input. Also, doing it this way a user can repeatedly click the button and get a different random number.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<link rel="stylesheet" href="css/main.css">
<title>Let's Make a Function</title>
</head>
<body>
<div class="container">
<h1>Let's Make a Function</h1>
<table width="300" cellpadding="10" border="0">
<tr><td>1st number:</td><td width="200"><input type="text" id="num1" size="6" onKeydown="Javascript: if (event.keyCode==13) getUserInput()" /></td></tr>
<tr><td>2nd number:</td><td><input type="text" id="num2" size="6" onKeydown="Javascript: if (event.keyCode==13) getUserInput()" /></td></tr>
<tr><td>Output:</td><td id="output"></td></tr>
<tr><td colspan="2"><button onclick="getUserInput();">Get Random Number</button></td></tr>
</table>
<script>
var number1;
var number2;
var result;
function randomNumber() {
if (isNaN(number1) || isNaN(number2)) {
result = "you didn't enter enough numbers";
} else if (number1 == number2) {
result = "You typed the same number.";
} else if (number1 > number2) {
result = Math.floor(Math.random() * (number1 - number2 + 1)) + number2;
} else if (number1 < number2) {
result = Math.floor(Math.random() * (number2 - number1 + 1)) + number1;
}
document.getElementById("output").innerHTML = result;
}
function getUserInput() {
number1= parseInt(document.getElementById("num1").value);
number2= parseInt(document.getElementById("num2").value);
randomNumber();
}
</script>
</div>
</body>
</html>
Happy coding. Thanks for the challenge! It's been a while.
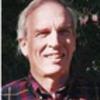
jcorum
71,830 PointsP.S., there's lots that purists would scoff at, in addition to the inclusion of the script in the html, rather than being a separate page: the use of global variables and the use of a table element instead of fancy positioning, for starters. But I'm sure there are more.

jessicawynn2
7,971 PointsThank you! It's definitely helpful to see it in a different way.