Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial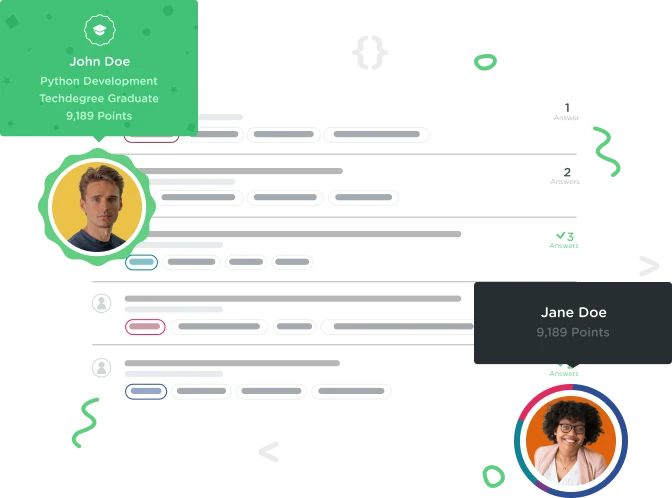

Michelle Brecunier
3,788 PointsBetter way to write this where the order of high and low can be switched?
I wanted to write a program where it doesn't matter if the second number the user inputs is lower or higher than the first. Is there a simpler/more elegant way to write this? This code seemed to work in the browser as far as I have tested it. I am also not sure what should happen if the values are equal.
var usernumber1 = parseInt(prompt('Enter a number:'));
var usernumber2 = parseInt(prompt('And another, please:'));
if (usernumber1 > usernumber2) {
alert(Math.floor(Math.random() * usernumber1) + usernumber2)};
if (usernumber2 > usernumber1) {
alert(Math.floor(Math.random() * usernumber2) + usernumber1)};

Michelle Brecunier
3,788 PointsI want to return a random number that is between the two numbers collected from the user, but I want the user able to enter a high number first and a low number second or the other way around while still have the random number come out correctly.
1 Answer

heidiatwood
6,013 PointsYes, you can use a couple of other Math objects that have not yet been covered in the course - Math.max and Math.min - to define a variable for the higher and lower numbers. This is how I would do that:
var userNumberA = parseInt(prompt("Enter a number."));
var userNumberB = parseInt(prompt("Enter another number"));
var higher = Math.max(userNumberA,userNumberB);
var lower = Math.min(userNumberA,userNumberB);
alert(Math.floor(Math.random() * (higher - lower)));
One caveat is that this method did not work when I included a number in my first two var names. (this is the first time I've tried to paste code into the forums, so bear with me if it's not correctly formatted ...)
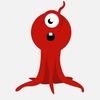
Justin Kraft
26,327 PointsIn your last alert message, simply add the lower number to get a number between the two:
var numberMin = parseFloat(prompt("Enter a number: "));
var numberMax = parseFloat(prompt("Enter a second number: "));
if(numberMax < numberMin) {
var temp = numberMax;
numberMax = numberMin;
numberMin = temp;
}
var newNumber = Math.floor(Math.random() * (numberMax - numberMin)) + numberMin;
document.write("A number between " + numberMin + " and " + numberMax + " is: " + newNumber);
Jacob Mishkin
23,118 PointsJacob Mishkin
23,118 PointsWhat are you trying to do? from the code presented it looks like you want to do is have a user input a number then randomize a number added by the second number. is that right?
if you want to change your code a bit, you could remove the second conditional statement then use an else clause, as the second conditional statement is redundant.