Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial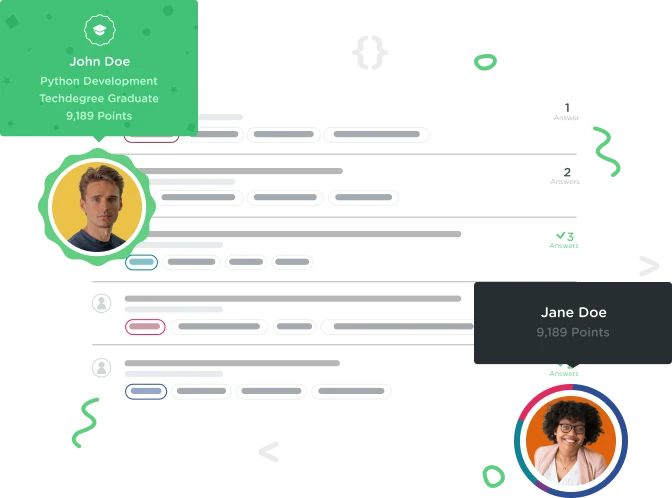

loxton
237 PointsBig O Notation Question. Why is this O(N)?
Why is the following expression O(N) and not O(logN)?
count = 0;
int i = N;
while (i > 1) {
count++;
i = i - 2;
}
i = N;
while (i > 1) {
count++;
i = i/2;
}
1 Answer

Yodit Nigusse
6,792 PointsWhen N is 10:
count-1: 5
count-2: 3
log(N): 3
When N is 100:
count-1: 50
count-2: 6
log(N): 6
When N is 100,000:
count-1: 50,000
count-2: 16
log(N): 16
The number of iteration for the first code is just half N. But for the second code, it must be O(log(N)). Subtracting 2 on every iteration would take at least half N to finish. But dividing by two on every iteration would take log(N) run.
If you are wondering how the maths work:
lets write an equation for how many times should you divide N to reach one:
1 = N/2^x
we are looking for x which tells us how many divisions we have made. So, lets rearrange the equation above:
2^x = N
x = log2(N) .... taking the logarithm of both sides.