Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial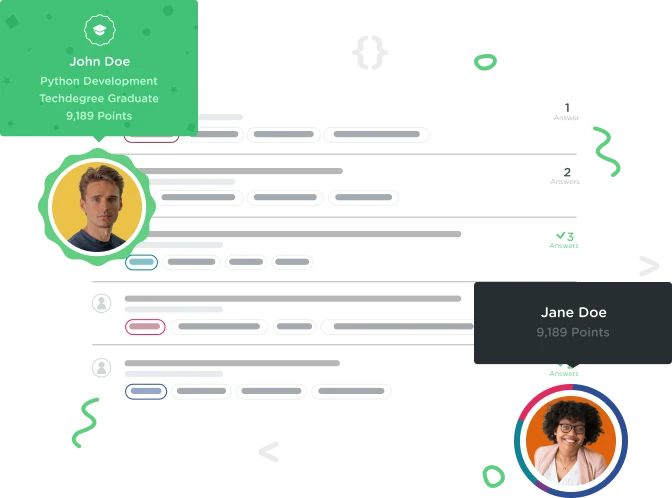

Tadiwanashe Dauya
3,864 PointsBind an ImageView member variable. Name it mMovieImage and again use the ID from the layout fi
Let's bind one more view. Bind an ImageView member variable. Name it mMovieImage and again use the ID from the layout file for the ImageView.
Bummer: There is a compiler error. Please click on preview to view your syntax errors!
MovieActivity.java
activity_movie.xml
import android.os.Bundle;
import android.view.View;
import android.widget.TextView;
import android.widget.ImageView;
ā
public class MovieActivity extends Activity {
@BindView(R.id.movieTitle) TextView mTitleLabel;
@InjectView(R.id.movieImage) ImageView mMovieImage;
ā
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_movie);
ButterKnife.bind(this);
ā
// The rest of the code is omitted for brevity :)
ButterKnife.inject(this);
}
}
ā
import android.os.Bundle;
import android.view.View;
import android.widget.TextView;
import android.widget.ImageView;
public class MovieActivity extends Activity {
@BindView(R.id.movieTitle) TextView mTitleLabel;
@InjectView(R.id.movieImage) ImageView mMovieImage;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_movie);
ButterKnife.bind(this);
// The rest of the code is omitted for brevity :)
ButterKnife.inject(this);
}
}
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/white" >
<TextView
android:id="@+id/movieTitle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="32sp"
android:text=""
android:layout_centerHorizontal="true"
android:layout_centerVertical="true" />
<ImageView
android:id="@+id/movieImage"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_above="@id/movieTitle" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/movieTitle"
android:layout_centerHorizontal="true"
android:id="@+id/linearLayout">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text=""
android:id="@+id/textView"
android:layout_weight="1"
android:gravity="left" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text=""
android:id="@+id/textView2"
android:layout_weight="1"
android:gravity="right" />
</LinearLayout>
</RelativeLayout>
1 Answer
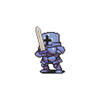
Judah Devasahayam
Full Stack JavaScript Techdegree Graduate 22,610 PointsYou're using InjectView
. Inject was changed to Bind so you're supposed to use BindView
. This is how your code should look like (not much difference just replace InjectView
with BindView
):
import android.os.Bundle;
import android.view.View;
import android.widget.TextView;
import android.widget.ImageView;
public class MovieActivity extends Activity {
@BindView(R.id.movieTitle) TextView mTitleLabel;
@BindView(R.id.movieImage) ImageView mMovieImage;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_movie);
ButterKnife.bind(this);
// The rest of the code is omitted for brevity :)
}
}