Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial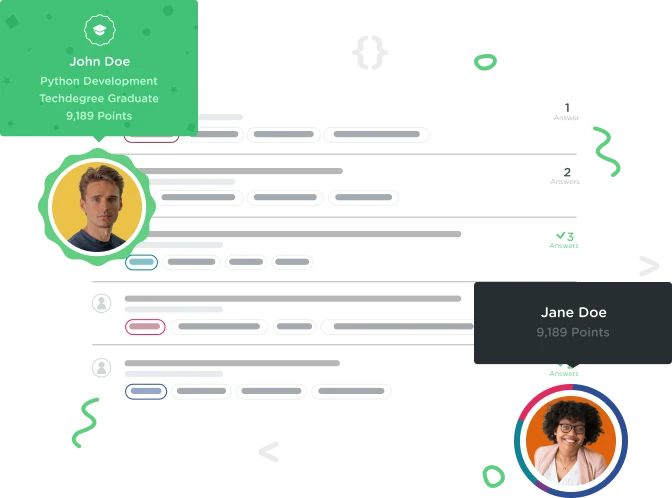

anonymous123
5,794 PointsBinding in JavaScript
Can someone explain binding ? I am taking the ES6 JavaScript Course and I want to understand what binding means because it is talked about in the MDN doc about arrow functions. I tried to find a doc specifically for binding but couldnt find one.
also do i have to know what the this keyword does right now? or will i be taught about in another JS course
βArrow functions do not have an own arguments binding in their scope; no arguments object is created when calling them.β What do you guys know about arrow functions not having their own arguments? Should I stop using them?
2 Answers
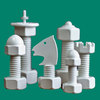
Steven Parker
231,269 PointsArrow functions provide a convenient and more compact way to define functions, and are particularly handy for callbacks. Just don't expect "this" to behave the same way in them as it does in other functions (once you learn how to use it!).
And "binding" just refers to the association of an identifier (name) with specific data. They are just warning you that the words "this" and "arguments" don't mean what you might expect inside arrow functions.
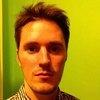
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsHere are the MDN docs for the bind() function.
This function is closely associate with .call()
and .apply()
, and there's a good tutorial about them here.
People used to have to deal with this stuff all the time, but luckily arrow functions have made a lot of this obsolete. For example, here's an example in the React docs.
They have this class method:
handleClick() {
this.setState(state => ({
isToggleOn: !state.isToggleOn
}));
}
But because it's NOT an arrow function, it's a class method, they have to call .bind() on it before they can pass it down as an event handler to the child element. Otherwise the this
would become undefined. They're doing this work in the constructor, but you also used to see it done right as we're passing it into the event handler: <button onClick={this.handleClick.bind(this)}>
. Luckily, we can use arrow functions now. You can even assign an arrow function to a class property rather than using class method. So the example in the React docs from above would be this now:
handleClick = () => {
this.setState(state => ({
isToggleOn: !state.isToggleOn
}));
}
...and you wouldn't have to call .bind().
I use arrow functions probably 99% of the time. I can't remember the last time I really needed one of the classic functions, and it avoids so many headaches with this
.
this
is an important concept. I'm not sure where you are in which course/track but I'm sure you will encounter some explanation of it sooner or later if you're following the prerequisites in order for the courses.