Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial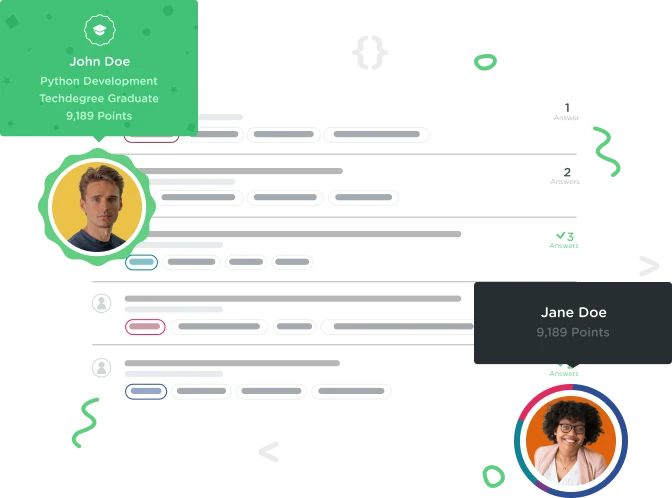
Shani Rivers
14,920 Pointsbin/rake spec uncovers TodoListsController.rb test failures
I am trying to figure out why this file fails, but I am not uncovering a reason, but perhaps its just me. I type in the bin/rake spec, the TodoListsController.rb file fails and I looked into the rows that it mentioned but I'm not seeing the issues. So I decided to comment out the whole file and it doesn't error out when I do bin/rake spec in the command line.
I am really trying to figure this out, but its just not making sense to me, since we didn't edit this file at all.
Any insight would be appreciated!
require 'spec_helper'
# This spec was generated by rspec-rails when you ran the scaffold generator.
# It demonstrates how one might use RSpec to specify the controller code that
# was generated by Rails when you ran the scaffold generator.
#
# It assumes that the implementation code is generated by the rails scaffold
# generator. If you are using any extension libraries to generate different
# controller code, this generated spec may or may not pass.
#
# It only uses APIs available in rails and/or rspec-rails. There are a number
# of tools you can use to make these specs even more expressive, but we're
# sticking to rails and rspec-rails APIs to keep things simple and stable.
#
# Compared to earlier versions of this generator, there is very limited use of
# stubs and message expectations in this spec. Stubs are only used when there
# is no simpler way to get a handle on the object needed for the example.
# Message expectations are only used when there is no simpler way to specify
# that an instance is receiving a specific message.
describe TodoListsController do
# This should return the minimal set of attributes required to create a valid
# TodoList. As you add validations to TodoList, be sure to
# adjust the attributes here as well.
let(:valid_attributes) { { "title" => "MyString" } }
# This should return the minimal set of values that should be in the session
# in order to pass any filters (e.g. authentication) defined in
# TodoListsController. Be sure to keep this updated too.
let(:valid_session) { {} }
describe "GET index" do
it "assigns all todo_lists as @todo_lists" do
todo_list = TodoList.create! valid_attributes
get :index, {}, valid_session
assigns(:todo_lists).should eq([todo_list])
end
end
describe "GET show" do
it "assigns the requested todo_list as @todo_list" do
todo_list = TodoList.create! valid_attributes
get :show, {:id => todo_list.to_param}, valid_session
assigns(:todo_list).should eq(todo_list)
end
end
describe "GET new" do
it "assigns a new todo_list as @todo_list" do
get :new, {}, valid_session
assigns(:todo_list).should be_a_new(TodoList)
end
end
describe "GET edit" do
it "assigns the requested todo_list as @todo_list" do
todo_list = TodoList.create! valid_attributes
get :edit, {:id => todo_list.to_param}, valid_session
assigns(:todo_list).should eq(todo_list)
end
end
describe "POST create" do
describe "with valid params" do
it "creates a new TodoList" do
expect {
post :create, {:todo_list => valid_attributes}, valid_session
}.to change(TodoList, :count).by(1)
end
it "assigns a newly created todo_list as @todo_list" do
post :create, {:todo_list => valid_attributes}, valid_session
assigns(:todo_list).should be_a(TodoList)
assigns(:todo_list).should be_persisted
end
it "redirects to the created todo_list" do
post :create, {:todo_list => valid_attributes}, valid_session
response.should redirect_to(TodoList.last)
end
end
describe "with invalid params" do
it "assigns a newly created but unsaved todo_list as @todo_list" do
# Trigger the behavior that occurs when invalid params are submitted
TodoList.any_instance.stub(:save).and_return(false)
post :create, {:todo_list => { "title" => "invalid value" }}, valid_session
assigns(:todo_list).should be_a_new(TodoList)
end
it "re-renders the 'new' template" do
# Trigger the behavior that occurs when invalid params are submitted
TodoList.any_instance.stub(:save).and_return(false)
post :create, {:todo_list => { "title" => "invalid value" }}, valid_session
response.should render_template("new")
end
end
end
describe "PUT update" do
describe "with valid params" do
it "updates the requested todo_list" do
todo_list = TodoList.create! valid_attributes
# Assuming there are no other todo_lists in the database, this
# specifies that the TodoList created on the previous line
# receives the :update_attributes message with whatever params are
# submitted in the request.
TodoList.any_instance.should_receive(:update).with({ "title" => "MyString" })
put :update, {:id => todo_list.to_param, :todo_list => { "title" => "MyString" }}, valid_session
end
it "assigns the requested todo_list as @todo_list" do
todo_list = TodoList.create! valid_attributes
put :update, {:id => todo_list.to_param, :todo_list => valid_attributes}, valid_session
assigns(:todo_list).should eq(todo_list)
end
it "redirects to the todo_list" do
todo_list = TodoList.create! valid_attributes
put :update, {:id => todo_list.to_param, :todo_list => valid_attributes}, valid_session
response.should redirect_to(todo_list)
end
end
describe "with invalid params" do
it "assigns the todo_list as @todo_list" do
todo_list = TodoList.create! valid_attributes
# Trigger the behavior that occurs when invalid params are submitted
TodoList.any_instance.stub(:save).and_return(false)
put :update, {:id => todo_list.to_param, :todo_list => { "title" => "invalid value" }}, valid_session
assigns(:todo_list).should eq(todo_list)
end
it "re-renders the 'edit' template" do
todo_list = TodoList.create! valid_attributes
# Trigger the behavior that occurs when invalid params are submitted
TodoList.any_instance.stub(:save).and_return(false)
put :update, {:id => todo_list.to_param, :todo_list => { "title" => "invalid value" }}, valid_session
response.should render_template("edit")
end
end
end
describe "DELETE destroy" do
it "destroys the requested todo_list" do
todo_list = TodoList.create! valid_attributes
expect {
delete :destroy, {:id => todo_list.to_param}, valid_session
}.to change(TodoList, :count).by(-1)
end
it "redirects to the todo_lists list" do
todo_list = TodoList.create! valid_attributes
delete :destroy, {:id => todo_list.to_param}, valid_session
response.should redirect_to(todo_lists_url)
end
end
end
Shani Rivers
14,920 PointsSorry about that, Maciej, I have edited my question.
1 Answer
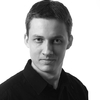
Maciej Czuchnowski
36,441 PointsOK, this line:
let(:valid_attributes) { { "title" => "MyString" } }
Defines the attributes that are given to an object at each test. From what I remember, your error said that the description was too short, because you have a validation that requires the description attribute to be at least 4 characters long. The line above only assigns a value to the title, so the description remains empty, that causes your error. Just add stuff to the valid_attributes, for example:
let(:valid_attributes) { { "title" => "MyString", "description" => "MyDescription" } }
It should do the trick unless you have other validations as well - adapt this line as necessary.
Shani Rivers
14,920 PointsThanks, Maciej! That corrected all the failures I was seeing! Now on to studying up on valid_attributes...
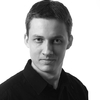
Maciej Czuchnowski
36,441 PointsIt's just a method created to DRY the code. You could instead do the whole List.create and list all the attribute in EVERY test, but then if you decide to change something, you'd have to change it in all places. Now you only do it in one place. It can be named anything you like.
Shani Rivers
14,920 PointsGood to know... I really appreciate your insight and help, of course. I would like to keep it as DRY as possible.
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsPlease include the source code of the controller spec that causes failures.