Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial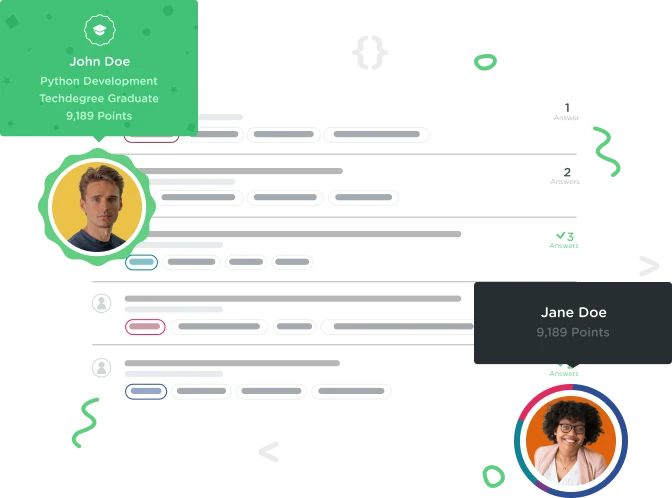

Preston Bennett
3,752 PointsBird moves with idle animation?
When the game starts, the bird follows the player just fine, however he is using the idle animation. I assume it has something to do with passing the velocity to the animator? here is my code:
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.AI;
public class BirdMovement : MonoBehaviour {
[SerializeField]
private Transform target;
private NavMeshAgent birdAgent;
private Animator birdAnimator;
// Use this for initialization
void Start () {
birdAgent = GetComponent<NavMeshAgent>();
birdAnimator = GetComponent<Animator>();
}
// Update is called once per frame
void Update () {
birdAgent.SetDestination(target.position);
float speed = birdAgent.velocity.magnitude;
birdAnimator.SetFloat("speed", speed);
}
}
I realize that the first two usings are unnecessary, but they were there to begin with so I left them (in case they will be necessary later, I don't know what I'm doing).
Here is the bird's inspector:
speed 5 angular speed 720 acceleration 8 stopping distance 5 auto breaking enabled
radius 1 height 4 high quality priority 50
here is the terrain's navigation:
agent radius 1 agent height 4 max slope 45 stop height 3
THANK YOU SO MUCH FOR ANY HELP!
1 Answer
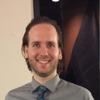
Andrew Haley
3,945 PointsI don't have my code in front of me but check the Animator settings on your bird prefab and cross-compare with your code, make sure your variable "speed" is named correctly, check for capitalization. It sounds like the transition from the idle animation isn't kicking off in the animator and this is likely due to not receiving the value it needs.

Preston Bennett
3,752 PointsThank you so much. Works perfect. Pesky little capital S. :)
Preston Bennett
3,752 PointsPreston Bennett
3,752 Pointssorry for the format, it was different when I wrote the question in the field provided. I didn't realize they would alter it.