Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial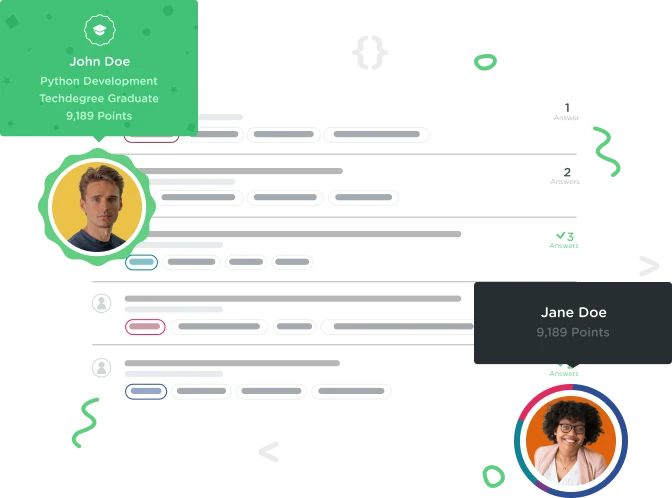
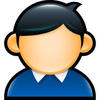
Daniel Hildreth
16,170 PointsBird Search In Action Error
So I am following the video in the Bird Search In Action within the Querying with LINQ course. I got a source error for my code when I tried to compile. Below are my Program.cs and BirdSearchExtension.cs:
Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace BirdWatcher
{
public class Program
{
public static void Main(string[] args)
{
var searchParameters = new BirdSearch
{
Size = "Medium",
Country = "Unnited States",
Colors = new List<string> { "White", "Brown", "Black" },
Page = 0,
PageSize = 5
};
Console.WriteLine("Type any key to begin search");
var birds = BirdRepository.LoadBirds();
while(Console.ReadKey().KeyChar != 'q')
{
Console.WriteLine($"Page: {searchParameters.Page}");
birds.Search(searchParameters).ToList().ForEach(b =>
{
Console.WriteLine($"CommonName: {b.CommonName}");
});
searchParameters.Page++;
}
}
}
}
BirdSearchExtension.cs
using System.Collections.Generic;
using System.Linq;
namespace BirdWatcher
{
public class BirdSearch
{
public string CommonName { get; set; }
public List<string> Colors { get; set; }
public string Country { get; set; }
public string Size { get; set; }
public int Page { get; set; }
public int PageSize { get; set; }
}
public static class BirdSearchExtension
{
public static IEnumerable<Bird> Search(this IEnumerable<Bird> source, Birdsearch search)
{
return source.Where(s => search.CommonName == null || s.CommonName.Contains(search.CommonName))
.Where(s => search.Country == null || s.Habitats.Any(h => h.Country.Contains(search.Country)))
.Where(s => search.Size == null || b.Size == search.Size)
.Where(s => search.Colors.Any(c => c == s.PrimaryColor) ||
search.Colors.Any(c => c == s.SecondaryColor) ||
search.Colors.Join(s.TertiaryColors,
sc => sc,
tc => tc,
(sc, tc) => (sc)).Any())
.Skip(page * pageSize)
.Take(pageSize);
}
}
}
This is the compiler error. The first error where it talked about the readme.md, I commented out thinking that's what it meant by the readme.md errors, and that is how I got the second error list.
1)
treehouse:~/workspace$ mcs * .cs -out:Program.exe
error CS2015: Source file `BirdWatcher.dll' is a binary file and not a text file
readme.md(1,0): error CS1525: Unexpected symbol `The'
readme.md(5,15): error CS1040: Preprocessor directives must appear as the first non-whitespace character on a line
error CS2001: Source file `.cs' could not be found
Compilation failed: 4 error(s), 0 warnings
2) (After commenting out entire readme.md file)
treehouse:~/workspace$ mcs * .cs -out:Program.exe
error CS2015: Source file `BirdWatcher.dll' is a binary file and not a text file
readme.md(1,0): error CS1525: Unexpected symbol `<'
readme.md(6,15): error CS1040: Preprocessor directives must appear as the first non-whitespace character on a line
error CS2001: Source file `.cs' could not be found
Compilation failed: 4 error(s), 0 warnings
1 Answer
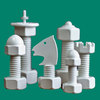
Steven Parker
231,268 Points
Your whole issue might be just a stray space.
Looking at your compile command:
treehouse:~/workspace$ mcs * .cs -out:Program.exe
I noticed you have a space between the asterisk "*
" and the ".cs
". You probably intended to write "*.cs
" which is a wildcard sequence that means "every file with a name that ends with .cs". But with the space it means "every file with a conventional name, plus one named .cs" (almost certainly not what you intended).