Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial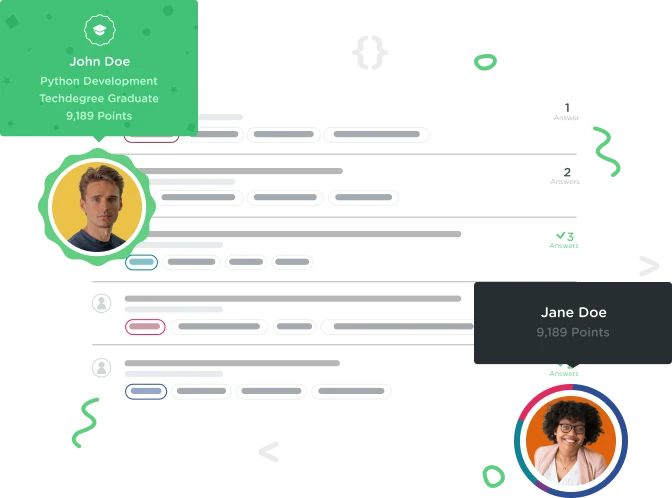

Evie Moore
1,030 PointsBird will not go into movement slot
I cannot not add the bird to bird movement on the utility page. I have wrote out the code 4 times now and the bird will not go into its slot. the game state text, player, and camera have all worked but the bird is my hold up. Any help would be great.
using UnityEngine;
using System.Collections;
using UnityEngine.UI;
public class GameState : MonoBehaviour {
private bool gameStarted = false;
[SerializeField]
private Text gameStateText;
[SerializeField]
private GameObject player;
[SerializeField]
private BirdMovement birdMovement;
[SerializeField]
private FollowCamera followCamera;
private float restartDelay = 3f;
private float restartTimer;
private PlayerMovement playerMovement;
private PlayerHealth playerHealth;
// Use this for initialization
void Start () {
Cursor.visible = false;
playerMovement = player.GetComponent<PlayerMovement> ();
playerHealth = player.GetComponent<PlayerHealth> ();
// prevent player movement
playerMovement.enabled = false;
// prevent bird movement
birdMovement.enabled = false;
// prevent the camera from moving
followCamera.enabled = false;
}
// Update is called once per frame
void Update () {
if (gameStarted == false && Input.GetKeyUp (KeyCode.Space)) {
StartGame();
}
if (playerHealth.alive == false) {
EndGame();
restartTimer = restartTimer + Time.deltaTime;
if (restartTimer >= restartDelay){
Application.LoadLevel (Application.loadedLevel);
}
}
}
private void StartGame() {
gameStarted = true;
gameStateText.color = Color.clear;
playerMovement.enabled = true;
birdMovement.enabled = true;
followCamera.enabled = true;
}
private void EndGame() {
gameStarted = false;
gameStateText.color = Color.white;
gameStateText.text = "Game Over!";
player.SetActive (false);
}
}
7 Answers
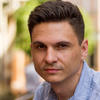
Nick Pettit
Treehouse TeacherHi Evie,
At first glance this code looks correct to me. To drop the Bird game object into the BirdMovement
slot in the inspector, the types need to match.
In this script, these are the two important lines to make that happen:
[SerializeField]
private BirdMovement birdMovement;
You've written these correctly in your script above, so my guess is that when you created the BirdMovement.cs script, something went wrong. Perhaps there's a typo in the name of the class, or maybe you named the class Birdmovement
instead of BirdMovement
which is different, since C# is a case sensitive language.
If that still doesn't fix it, post your BirdMovement script here and we'll keep working to figure it out together.

Evie Moore
1,030 PointsI thought it might have been in the other script as well but the strange thing is that when you hit the circle next to it nothing at all comes up to go in the slot. I did grab the BirdMovement script to post here.
using UnityEngine;
using System.Collections;
public class BirdMovement : MonoBehaviour {
[SerializeField]
private Transform target;
private NavMeshAgent birdAgent;
private Animator birdAnimator;
// Use this for initialization
void Start () {
birdAgent = GetComponent<NavMeshAgent> ();
birdAnimator = GetComponent<Animator> ();
}
// Update is called once per frame
void Update () {
// Set the birds destination
birdAgent.SetDestination (target.position);
//,esure the mag of agents velocity
float speed = birdAgent.velocity.magnitude;
// pass the velocity to the animator component
birdAnimator.SetFloat ("Speed", speed);
}
}
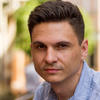
Nick Pettit
Treehouse TeacherHmmm... That's strange.
A few things to check and/or try:
- I'm guessing you've already done this, but just in case: The BirdMovement script needs to be attached to the Bird game object. This will allow you to drop the Bird game object onto the "Bird Movement" slot in the Inspector window on the GameState script and have it recognize the BirdMovement script attached to the Bird.
- Try restarting Unity. On rare occasion the compilation in the Unity editor can get into a strange state, but restarting it fixes it.
- Check the Console window. In the Default layout, it should be on the bottom left of the screen in a tab next to the Project window. If there are any errors in the Console, you'll often need to correct them in order for the Inspector to update in response to script changes. Double clicking any errors will usually bring you to the part of your script where there's a problem. If you don't understand the error, post it here and I can try to help.
If none of those things work, would you mind putting your entire project into a zip file, uploading it, and then posting the link to it here? (Dropbox usually works best for this.) That would help more than anything else.
Thanks for being patient! Patience to work through problems is a big part of programming. :)

Evie Moore
1,030 PointsSo if I am correct should i change [SerializeField] private BirdMovement birdMovement
to [SerializeField] private GameObject birdMovement; Is that how I turn the bird into a game object?
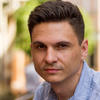
Nick Pettit
Treehouse TeacherHi Evie,
No. The code you posted looks correct to me. The bird is already a game object, but in the GameState
script, you're just looking for the BirdMovement
script that's attached to the bird game object.
Because the code looks correct, it either means something is wrong elsewhere in Unity, or there actually is an error in the script.
Do you think you could zip up your project and share the link here? That would be the fastest way for me to debug it.

Evie Moore
1,030 PointsI have never uploaded a zip file without email before so I choose this website to do it. I hope this one is ok and if not please let me know where you would like me to upload it. Thank you also for helping me on this.
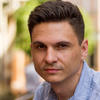
Nick Pettit
Treehouse TeacherHi Evie,
I took a look at this zip file, and it just looks like the assets that are included with the course.
What I need is your entire Unity project directory. Your Unity project is the folder you would have created at the start of the course when you first created the project. Hopefully that makes sense!

Evie Moore
1,030 PointsThis is the file so it should have what you are looking for. http://www.filedropper.com/frogsandlogscompletefile